mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
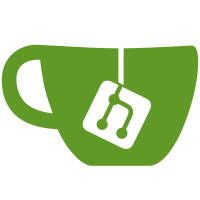
Small refactor to be able to use custom transports to call remote plugins. Signed-off-by: David Calavera <david.calavera@gmail.com>
36 lines
819 B
Go
36 lines
819 B
Go
package transport
|
|
|
|
import (
|
|
"io"
|
|
"net/http"
|
|
)
|
|
|
|
// httpTransport holds an http.RoundTripper
|
|
// and information about the scheme and address the transport
|
|
// sends request to.
|
|
type httpTransport struct {
|
|
http.RoundTripper
|
|
scheme string
|
|
addr string
|
|
}
|
|
|
|
// NewHTTPTransport creates a new httpTransport.
|
|
func NewHTTPTransport(r http.RoundTripper, scheme, addr string) Transport {
|
|
return httpTransport{
|
|
RoundTripper: r,
|
|
scheme: scheme,
|
|
addr: addr,
|
|
}
|
|
}
|
|
|
|
// NewRequest creates a new http.Request and sets the URL
|
|
// scheme and address with the transport's fields.
|
|
func (t httpTransport) NewRequest(path string, data io.Reader) (*http.Request, error) {
|
|
req, err := newHTTPRequest(path, data)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
req.URL.Scheme = t.scheme
|
|
req.URL.Host = t.addr
|
|
return req, nil
|
|
}
|