mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
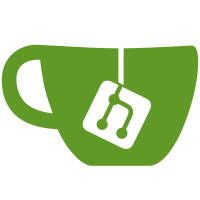
Remove forked reference package. Use normalized named values everywhere and familiar functions to convert back to familiar strings for UX and storage compatibility. Enforce that the source repository in the distribution metadata is always a normalized string, ignore invalid values which are not. Update distribution tests to use normalized values. Signed-off-by: Derek McGowan <derek@mcgstyle.net> (github: dmcgowan)
61 lines
1.9 KiB
Go
61 lines
1.9 KiB
Go
package client
|
|
|
|
import (
|
|
"io"
|
|
"net/http"
|
|
"net/url"
|
|
|
|
"golang.org/x/net/context"
|
|
|
|
"github.com/docker/distribution/reference"
|
|
"github.com/docker/docker/api/types"
|
|
)
|
|
|
|
// ImagePull requests the docker host to pull an image from a remote registry.
|
|
// It executes the privileged function if the operation is unauthorized
|
|
// and it tries one more time.
|
|
// It's up to the caller to handle the io.ReadCloser and close it properly.
|
|
//
|
|
// FIXME(vdemeester): there is currently used in a few way in docker/docker
|
|
// - if not in trusted content, ref is used to pass the whole reference, and tag is empty
|
|
// - if in trusted content, ref is used to pass the reference name, and tag for the digest
|
|
func (cli *Client) ImagePull(ctx context.Context, refStr string, options types.ImagePullOptions) (io.ReadCloser, error) {
|
|
ref, err := reference.ParseNormalizedNamed(refStr)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
query := url.Values{}
|
|
query.Set("fromImage", reference.FamiliarName(ref))
|
|
if !options.All {
|
|
query.Set("tag", getAPITagFromNamedRef(ref))
|
|
}
|
|
|
|
resp, err := cli.tryImageCreate(ctx, query, options.RegistryAuth)
|
|
if resp.statusCode == http.StatusUnauthorized && options.PrivilegeFunc != nil {
|
|
newAuthHeader, privilegeErr := options.PrivilegeFunc()
|
|
if privilegeErr != nil {
|
|
return nil, privilegeErr
|
|
}
|
|
resp, err = cli.tryImageCreate(ctx, query, newAuthHeader)
|
|
}
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return resp.body, nil
|
|
}
|
|
|
|
// getAPITagFromNamedRef returns a tag from the specified reference.
|
|
// This function is necessary as long as the docker "server" api expects
|
|
// digests to be sent as tags and makes a distinction between the name
|
|
// and tag/digest part of a reference.
|
|
func getAPITagFromNamedRef(ref reference.Named) string {
|
|
if digested, ok := ref.(reference.Digested); ok {
|
|
return digested.Digest().String()
|
|
}
|
|
ref = reference.TagNameOnly(ref)
|
|
if tagged, ok := ref.(reference.Tagged); ok {
|
|
return tagged.Tag()
|
|
}
|
|
return ""
|
|
}
|