mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
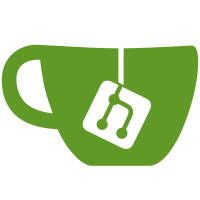
The `archive` package defines aliases for `io.ReadCloser` and `io.Reader`. These don't seem to provide an benefit other than type decoration. Per this change, several unnecessary type cases were removed. Signed-off-by: Stephen J Day <stephen.day@docker.com>
60 lines
1.6 KiB
Go
60 lines
1.6 KiB
Go
package daemon
|
|
|
|
import (
|
|
"fmt"
|
|
"io"
|
|
"runtime"
|
|
|
|
"github.com/docker/docker/container"
|
|
"github.com/docker/docker/pkg/archive"
|
|
"github.com/docker/docker/pkg/ioutils"
|
|
)
|
|
|
|
// ContainerExport writes the contents of the container to the given
|
|
// writer. An error is returned if the container cannot be found.
|
|
func (daemon *Daemon) ContainerExport(name string, out io.Writer) error {
|
|
if runtime.GOOS == "windows" {
|
|
return fmt.Errorf("the daemon on this platform does not support export of a container")
|
|
}
|
|
|
|
container, err := daemon.GetContainer(name)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
|
|
data, err := daemon.containerExport(container)
|
|
if err != nil {
|
|
return fmt.Errorf("Error exporting container %s: %v", name, err)
|
|
}
|
|
defer data.Close()
|
|
|
|
// Stream the entire contents of the container (basically a volatile snapshot)
|
|
if _, err := io.Copy(out, data); err != nil {
|
|
return fmt.Errorf("Error exporting container %s: %v", name, err)
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (daemon *Daemon) containerExport(container *container.Container) (io.ReadCloser, error) {
|
|
if err := daemon.Mount(container); err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
uidMaps, gidMaps := daemon.GetUIDGIDMaps()
|
|
archive, err := archive.TarWithOptions(container.BaseFS, &archive.TarOptions{
|
|
Compression: archive.Uncompressed,
|
|
UIDMaps: uidMaps,
|
|
GIDMaps: gidMaps,
|
|
})
|
|
if err != nil {
|
|
daemon.Unmount(container)
|
|
return nil, err
|
|
}
|
|
arch := ioutils.NewReadCloserWrapper(archive, func() error {
|
|
err := archive.Close()
|
|
daemon.Unmount(container)
|
|
return err
|
|
})
|
|
daemon.LogContainerEvent(container, "export")
|
|
return arch, err
|
|
}
|