mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
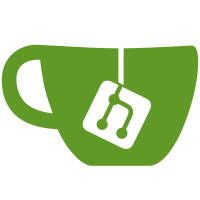
I noticed that we're using a homegrown package for assertions. The functions are extremely similar to testify, but with enough slight differences to be confusing (for example, Equal takes its arguments in a different order). We already vendor testify, and it's used in a few places by tests. I also found some problems with pkg/testutil/assert. For example, the NotNil function seems to be broken. It checks the argument against "nil", which only works for an interface. If you pass in a nil map or slice, the equality check will fail. In the interest of avoiding NIH, I'm proposing replacing pkg/testutil/assert with testify. The test code looks almost the same, but we avoid the confusion of having two similar but slightly different assertion packages, and having to maintain our own package instead of using a commonly-used one. In the process, I found a few places where the tests should halt if an assertion fails, so I've made those cases (that I noticed) use "require" instead of "assert", and I've vendored the "require" package from testify alongside the already-present "assert" package. Signed-off-by: Aaron Lehmann <aaron.lehmann@docker.com>
119 lines
2.8 KiB
Go
119 lines
2.8 KiB
Go
package client
|
|
|
|
import (
|
|
"bytes"
|
|
"encoding/json"
|
|
"fmt"
|
|
"io/ioutil"
|
|
"net/http"
|
|
"strings"
|
|
"testing"
|
|
|
|
"github.com/docker/docker/api/types"
|
|
"github.com/docker/docker/api/types/filters"
|
|
"github.com/stretchr/testify/assert"
|
|
"golang.org/x/net/context"
|
|
)
|
|
|
|
func TestImagesPruneError(t *testing.T) {
|
|
client := &Client{
|
|
client: newMockClient(errorMock(http.StatusInternalServerError, "Server error")),
|
|
version: "1.25",
|
|
}
|
|
|
|
filters := filters.NewArgs()
|
|
|
|
_, err := client.ImagesPrune(context.Background(), filters)
|
|
assert.EqualError(t, err, "Error response from daemon: Server error")
|
|
}
|
|
|
|
func TestImagesPrune(t *testing.T) {
|
|
expectedURL := "/v1.25/images/prune"
|
|
|
|
danglingFilters := filters.NewArgs()
|
|
danglingFilters.Add("dangling", "true")
|
|
|
|
noDanglingFilters := filters.NewArgs()
|
|
noDanglingFilters.Add("dangling", "false")
|
|
|
|
labelFilters := filters.NewArgs()
|
|
labelFilters.Add("dangling", "true")
|
|
labelFilters.Add("label", "label1=foo")
|
|
labelFilters.Add("label", "label2!=bar")
|
|
|
|
listCases := []struct {
|
|
filters filters.Args
|
|
expectedQueryParams map[string]string
|
|
}{
|
|
{
|
|
filters: filters.Args{},
|
|
expectedQueryParams: map[string]string{
|
|
"until": "",
|
|
"filter": "",
|
|
"filters": "",
|
|
},
|
|
},
|
|
{
|
|
filters: danglingFilters,
|
|
expectedQueryParams: map[string]string{
|
|
"until": "",
|
|
"filter": "",
|
|
"filters": `{"dangling":{"true":true}}`,
|
|
},
|
|
},
|
|
{
|
|
filters: noDanglingFilters,
|
|
expectedQueryParams: map[string]string{
|
|
"until": "",
|
|
"filter": "",
|
|
"filters": `{"dangling":{"false":true}}`,
|
|
},
|
|
},
|
|
{
|
|
filters: labelFilters,
|
|
expectedQueryParams: map[string]string{
|
|
"until": "",
|
|
"filter": "",
|
|
"filters": `{"dangling":{"true":true},"label":{"label1=foo":true,"label2!=bar":true}}`,
|
|
},
|
|
},
|
|
}
|
|
for _, listCase := range listCases {
|
|
client := &Client{
|
|
client: newMockClient(func(req *http.Request) (*http.Response, error) {
|
|
if !strings.HasPrefix(req.URL.Path, expectedURL) {
|
|
return nil, fmt.Errorf("Expected URL '%s', got '%s'", expectedURL, req.URL)
|
|
}
|
|
query := req.URL.Query()
|
|
for key, expected := range listCase.expectedQueryParams {
|
|
actual := query.Get(key)
|
|
assert.Equal(t, expected, actual)
|
|
}
|
|
content, err := json.Marshal(types.ImagesPruneReport{
|
|
ImagesDeleted: []types.ImageDeleteResponseItem{
|
|
{
|
|
Deleted: "image_id1",
|
|
},
|
|
{
|
|
Deleted: "image_id2",
|
|
},
|
|
},
|
|
SpaceReclaimed: 9999,
|
|
})
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return &http.Response{
|
|
StatusCode: http.StatusOK,
|
|
Body: ioutil.NopCloser(bytes.NewReader(content)),
|
|
}, nil
|
|
}),
|
|
version: "1.25",
|
|
}
|
|
|
|
report, err := client.ImagesPrune(context.Background(), listCase.filters)
|
|
assert.NoError(t, err)
|
|
assert.Len(t, report.ImagesDeleted, 2)
|
|
assert.Equal(t, uint64(9999), report.SpaceReclaimed)
|
|
}
|
|
}
|