mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
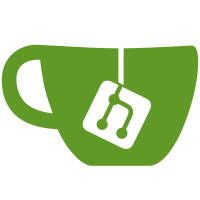
The jsonlog logger currently allows specifying envs and labels that should be propagated to the log message, however there has been no way to read that back. This adds a new API option to enable inserting these attrs back to the log reader. With timestamps, this looks like so: ``` 92016-04-08T15:28:09.835913720Z foo=bar,hello=world hello ``` The extra attrs are comma separated before the log message but after timestamps. Without timestaps it looks like so: ``` foo=bar,hello=world hello ``` Signed-off-by: Brian Goff <cpuguy83@gmail.com>
42 lines
1.2 KiB
Go
42 lines
1.2 KiB
Go
package jsonlog
|
|
|
|
import (
|
|
"encoding/json"
|
|
"fmt"
|
|
"time"
|
|
)
|
|
|
|
// JSONLog represents a log message, typically a single entry from a given log stream.
|
|
// JSONLogs can be easily serialized to and from JSON and support custom formatting.
|
|
type JSONLog struct {
|
|
// Log is the log message
|
|
Log string `json:"log,omitempty"`
|
|
// Stream is the log source
|
|
Stream string `json:"stream,omitempty"`
|
|
// Created is the created timestamp of log
|
|
Created time.Time `json:"time"`
|
|
// Attrs is the list of extra attributes provided by the user
|
|
Attrs map[string]string `json:"attrs,omitempty"`
|
|
}
|
|
|
|
// Format returns the log formatted according to format
|
|
// If format is nil, returns the log message
|
|
// If format is json, returns the log marshaled in json format
|
|
// By default, returns the log with the log time formatted according to format.
|
|
func (jl *JSONLog) Format(format string) (string, error) {
|
|
if format == "" {
|
|
return jl.Log, nil
|
|
}
|
|
if format == "json" {
|
|
m, err := json.Marshal(jl)
|
|
return string(m), err
|
|
}
|
|
return fmt.Sprintf("%s %s", jl.Created.Format(format), jl.Log), nil
|
|
}
|
|
|
|
// Reset resets the log to nil.
|
|
func (jl *JSONLog) Reset() {
|
|
jl.Log = ""
|
|
jl.Stream = ""
|
|
jl.Created = time.Time{}
|
|
}
|