mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
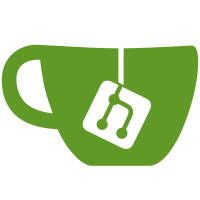
`fuse-overlayfs` provides rootless overlayfs functionality without depending on any kernel patch. Aside from rootless, `fuse-overlayfs` could be potentially used for eliminating `chown()` calls that happen in userns-remap mode, because `fuse-overlayfs` also provides shiftfs functionality. System requirements: * fuse-overlayfs needs to be installed. Tested with 0.7.6. * kernel >= 4.18 Unit test: `go test -exec sudo -v ./daemon/graphdriver/fuse-overlayfs` The implementation is based on Podman's `overlay` driver which supports both kernel-mode overlayfs and fuse-overlayfs in the single driver instance: https://github.com/containers/storage/blob/39a8d5ed/drivers/overlay/overlay.go However, Moby's implementation aims to decouple `fuse-overlayfs` driver from the kernel-mode driver (`overlay2`) for simplicity. Fix #40218 Signed-off-by: Akihiro Suda <akihiro.suda.cz@hco.ntt.co.jp>
85 lines
2.2 KiB
Go
85 lines
2.2 KiB
Go
// +build linux
|
|
|
|
package fuseoverlayfs // import "github.com/docker/docker/daemon/graphdriver/fuse-overlayfs"
|
|
|
|
import (
|
|
"testing"
|
|
|
|
"github.com/docker/docker/daemon/graphdriver"
|
|
"github.com/docker/docker/daemon/graphdriver/graphtest"
|
|
"github.com/docker/docker/pkg/archive"
|
|
"github.com/docker/docker/pkg/reexec"
|
|
)
|
|
|
|
func init() {
|
|
// Do not sure chroot to speed run time and allow archive
|
|
// errors or hangs to be debugged directly from the test process.
|
|
untar = archive.UntarUncompressed
|
|
graphdriver.ApplyUncompressedLayer = archive.ApplyUncompressedLayer
|
|
|
|
reexec.Init()
|
|
}
|
|
|
|
// This avoids creating a new driver for each test if all tests are run
|
|
// Make sure to put new tests between TestFUSEOverlayFSSetup and TestFUSEOverlayFSTeardown
|
|
func TestFUSEOverlayFSSetup(t *testing.T) {
|
|
graphtest.GetDriver(t, driverName)
|
|
}
|
|
|
|
func TestFUSEOverlayFSCreateEmpty(t *testing.T) {
|
|
graphtest.DriverTestCreateEmpty(t, driverName)
|
|
}
|
|
|
|
func TestFUSEOverlayFSCreateBase(t *testing.T) {
|
|
graphtest.DriverTestCreateBase(t, driverName)
|
|
}
|
|
|
|
func TestFUSEOverlayFSCreateSnap(t *testing.T) {
|
|
graphtest.DriverTestCreateSnap(t, driverName)
|
|
}
|
|
|
|
func TestFUSEOverlayFS128LayerRead(t *testing.T) {
|
|
graphtest.DriverTestDeepLayerRead(t, 128, driverName)
|
|
}
|
|
|
|
func TestFUSEOverlayFSTeardown(t *testing.T) {
|
|
graphtest.PutDriver(t)
|
|
}
|
|
|
|
// Benchmarks should always setup new driver
|
|
|
|
func BenchmarkExists(b *testing.B) {
|
|
graphtest.DriverBenchExists(b, driverName)
|
|
}
|
|
|
|
func BenchmarkGetEmpty(b *testing.B) {
|
|
graphtest.DriverBenchGetEmpty(b, driverName)
|
|
}
|
|
|
|
func BenchmarkDiffBase(b *testing.B) {
|
|
graphtest.DriverBenchDiffBase(b, driverName)
|
|
}
|
|
|
|
func BenchmarkDiffSmallUpper(b *testing.B) {
|
|
graphtest.DriverBenchDiffN(b, 10, 10, driverName)
|
|
}
|
|
|
|
func BenchmarkDiff10KFileUpper(b *testing.B) {
|
|
graphtest.DriverBenchDiffN(b, 10, 10000, driverName)
|
|
}
|
|
|
|
func BenchmarkDiff10KFilesBottom(b *testing.B) {
|
|
graphtest.DriverBenchDiffN(b, 10000, 10, driverName)
|
|
}
|
|
|
|
func BenchmarkDiffApply100(b *testing.B) {
|
|
graphtest.DriverBenchDiffApplyN(b, 100, driverName)
|
|
}
|
|
|
|
func BenchmarkDiff20Layers(b *testing.B) {
|
|
graphtest.DriverBenchDeepLayerDiff(b, 20, driverName)
|
|
}
|
|
|
|
func BenchmarkRead20Layers(b *testing.B) {
|
|
graphtest.DriverBenchDeepLayerRead(b, 20, driverName)
|
|
}
|