mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
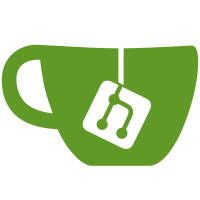
Move plugins to shared distribution stack with images. Create immutable plugin config that matches schema2 requirements. Ensure data being pushed is same as pulled/created. Store distribution artifacts in a blobstore. Run init layer setup for every plugin start. Fix breakouts from unsafe file accesses. Add support for `docker plugin install --alias` Uses normalized references for default names to avoid collisions when using default hosts/tags. Some refactoring of the plugin manager to support the change, like removing the singleton manager and adding manager config struct. Signed-off-by: Tonis Tiigi <tonistiigi@gmail.com> Signed-off-by: Derek McGowan <derek@mcgstyle.net>
55 lines
1.3 KiB
Go
55 lines
1.3 KiB
Go
package plugin
|
|
|
|
import (
|
|
"fmt"
|
|
|
|
"github.com/docker/docker/api/types"
|
|
"github.com/docker/docker/cli"
|
|
"github.com/docker/docker/cli/command"
|
|
"github.com/spf13/cobra"
|
|
"golang.org/x/net/context"
|
|
)
|
|
|
|
type rmOptions struct {
|
|
force bool
|
|
|
|
plugins []string
|
|
}
|
|
|
|
func newRemoveCommand(dockerCli *command.DockerCli) *cobra.Command {
|
|
var opts rmOptions
|
|
|
|
cmd := &cobra.Command{
|
|
Use: "rm [OPTIONS] PLUGIN [PLUGIN...]",
|
|
Short: "Remove one or more plugins",
|
|
Aliases: []string{"remove"},
|
|
Args: cli.RequiresMinArgs(1),
|
|
RunE: func(cmd *cobra.Command, args []string) error {
|
|
opts.plugins = args
|
|
return runRemove(dockerCli, &opts)
|
|
},
|
|
}
|
|
|
|
flags := cmd.Flags()
|
|
flags.BoolVarP(&opts.force, "force", "f", false, "Force the removal of an active plugin")
|
|
return cmd
|
|
}
|
|
|
|
func runRemove(dockerCli *command.DockerCli, opts *rmOptions) error {
|
|
ctx := context.Background()
|
|
|
|
var errs cli.Errors
|
|
for _, name := range opts.plugins {
|
|
// TODO: pass names to api instead of making multiple api calls
|
|
if err := dockerCli.Client().PluginRemove(ctx, name, types.PluginRemoveOptions{Force: opts.force}); err != nil {
|
|
errs = append(errs, err)
|
|
continue
|
|
}
|
|
fmt.Fprintln(dockerCli.Out(), name)
|
|
}
|
|
// Do not simplify to `return errs` because even if errs == nil, it is not a nil-error interface value.
|
|
if errs != nil {
|
|
return errs
|
|
}
|
|
return nil
|
|
}
|