mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
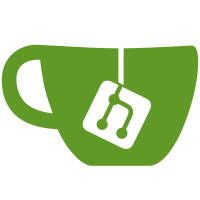
Start work on adding unit tests to our cli code in order to have to write less costly integration test. Signed-off-by: Vincent Demeester <vincent@sbr.pm>
44 lines
906 B
Go
44 lines
906 B
Go
package swarm
|
|
|
|
import (
|
|
"fmt"
|
|
|
|
"golang.org/x/net/context"
|
|
|
|
"github.com/docker/docker/cli"
|
|
"github.com/docker/docker/cli/command"
|
|
"github.com/spf13/cobra"
|
|
)
|
|
|
|
type leaveOptions struct {
|
|
force bool
|
|
}
|
|
|
|
func newLeaveCommand(dockerCli command.Cli) *cobra.Command {
|
|
opts := leaveOptions{}
|
|
|
|
cmd := &cobra.Command{
|
|
Use: "leave [OPTIONS]",
|
|
Short: "Leave the swarm",
|
|
Args: cli.NoArgs,
|
|
RunE: func(cmd *cobra.Command, args []string) error {
|
|
return runLeave(dockerCli, opts)
|
|
},
|
|
}
|
|
|
|
flags := cmd.Flags()
|
|
flags.BoolVarP(&opts.force, "force", "f", false, "Force this node to leave the swarm, ignoring warnings")
|
|
return cmd
|
|
}
|
|
|
|
func runLeave(dockerCli command.Cli, opts leaveOptions) error {
|
|
client := dockerCli.Client()
|
|
ctx := context.Background()
|
|
|
|
if err := client.SwarmLeave(ctx, opts.force); err != nil {
|
|
return err
|
|
}
|
|
|
|
fmt.Fprintln(dockerCli.Out(), "Node left the swarm.")
|
|
return nil
|
|
}
|