mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
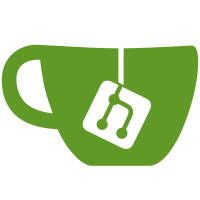
Docker-DCO-1.1-Signed-off-by: Michael Crosby <michael@crosbymichael.com> (github: crosbymichael)
59 lines
1.3 KiB
Go
59 lines
1.3 KiB
Go
package selinux_test
|
|
|
|
import (
|
|
"github.com/dotcloud/docker/pkg/selinux"
|
|
"os"
|
|
"testing"
|
|
)
|
|
|
|
func testSetfilecon(t *testing.T) {
|
|
if selinux.SelinuxEnabled() {
|
|
tmp := "selinux_test"
|
|
out, _ := os.OpenFile(tmp, os.O_WRONLY, 0)
|
|
out.Close()
|
|
err := selinux.Setfilecon(tmp, "system_u:object_r:bin_t:s0")
|
|
if err != nil {
|
|
t.Log("Setfilecon failed")
|
|
t.Fatal(err)
|
|
}
|
|
os.Remove(tmp)
|
|
}
|
|
}
|
|
|
|
func TestSELinux(t *testing.T) {
|
|
var (
|
|
err error
|
|
plabel, flabel string
|
|
)
|
|
|
|
if selinux.SelinuxEnabled() {
|
|
t.Log("Enabled")
|
|
plabel, flabel = selinux.GetLxcContexts()
|
|
t.Log(plabel)
|
|
t.Log(flabel)
|
|
plabel, flabel = selinux.GetLxcContexts()
|
|
t.Log(plabel)
|
|
t.Log(flabel)
|
|
t.Log("getenforce ", selinux.SelinuxGetEnforce())
|
|
t.Log("getenforcemode ", selinux.SelinuxGetEnforceMode())
|
|
pid := os.Getpid()
|
|
t.Log("PID:%d MCS:%s\n", pid, selinux.IntToMcs(pid, 1023))
|
|
err = selinux.Setfscreatecon("unconfined_u:unconfined_r:unconfined_t:s0")
|
|
if err == nil {
|
|
t.Log(selinux.Getfscreatecon())
|
|
} else {
|
|
t.Log("setfscreatecon failed", err)
|
|
t.Fatal(err)
|
|
}
|
|
err = selinux.Setfscreatecon("")
|
|
if err == nil {
|
|
t.Log(selinux.Getfscreatecon())
|
|
} else {
|
|
t.Log("setfscreatecon failed", err)
|
|
t.Fatal(err)
|
|
}
|
|
t.Log(selinux.Getpidcon(1))
|
|
} else {
|
|
t.Log("Disabled")
|
|
}
|
|
}
|