mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
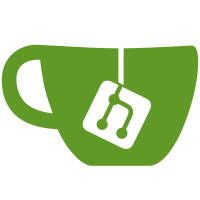
These settings need to be in the HostConfig so that they are not committed to an image and cannot introduce a security issue. We can safely move this field from the Config to the HostConfig without any regressions because these settings are consumed at container created and used to populate fields on the Container struct. Because of this, existing settings will be honored for containers already created on a daemon with custom security settings and prevent values being consumed via an Image. Signed-off-by: Michael Crosby <crosbymichael@gmail.com> Conflicts: daemon/create.go changing config to hostConfig was required to fix the build
39 lines
1.1 KiB
Go
39 lines
1.1 KiB
Go
package daemon
|
|
|
|
import (
|
|
"testing"
|
|
|
|
"github.com/docker/docker/runconfig"
|
|
)
|
|
|
|
func TestParseSecurityOpt(t *testing.T) {
|
|
container := &Container{}
|
|
config := &runconfig.HostConfig{}
|
|
|
|
// test apparmor
|
|
config.SecurityOpt = []string{"apparmor:test_profile"}
|
|
if err := parseSecurityOpt(container, config); err != nil {
|
|
t.Fatalf("Unexpected parseSecurityOpt error: %v", err)
|
|
}
|
|
if container.AppArmorProfile != "test_profile" {
|
|
t.Fatalf("Unexpected AppArmorProfile, expected: \"test_profile\", got %q", container.AppArmorProfile)
|
|
}
|
|
|
|
// test valid label
|
|
config.SecurityOpt = []string{"label:user:USER"}
|
|
if err := parseSecurityOpt(container, config); err != nil {
|
|
t.Fatalf("Unexpected parseSecurityOpt error: %v", err)
|
|
}
|
|
|
|
// test invalid label
|
|
config.SecurityOpt = []string{"label"}
|
|
if err := parseSecurityOpt(container, config); err == nil {
|
|
t.Fatal("Expected parseSecurityOpt error, got nil")
|
|
}
|
|
|
|
// test invalid opt
|
|
config.SecurityOpt = []string{"test"}
|
|
if err := parseSecurityOpt(container, config); err == nil {
|
|
t.Fatal("Expected parseSecurityOpt error, got nil")
|
|
}
|
|
}
|