mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
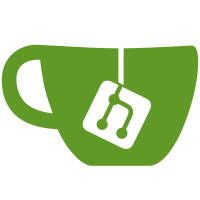
Although having a request ID available throughout the codebase is very valuable, the impact of requiring a Context as an argument to every function in the codepath of an API request, is too significant and was not properly understood at the time of the review. Furthermore, mixing API-layer code with non-API-layer code makes the latter usable only by API-layer code (one that has a notion of Context). This reverts commitde41640435
, reversing changes made to7daeecd42d
. Signed-off-by: Tibor Vass <tibor@docker.com> Conflicts: api/server/container.go builder/internals.go daemon/container_unix.go daemon/create.go
324 lines
10 KiB
Go
324 lines
10 KiB
Go
package daemon
|
|
|
|
import (
|
|
"errors"
|
|
"io"
|
|
"os"
|
|
"path/filepath"
|
|
"strings"
|
|
|
|
"github.com/docker/docker/api/types"
|
|
"github.com/docker/docker/pkg/archive"
|
|
"github.com/docker/docker/pkg/chrootarchive"
|
|
"github.com/docker/docker/pkg/ioutils"
|
|
)
|
|
|
|
// ErrExtractPointNotDirectory is used to convey that the operation to extract
|
|
// a tar archive to a directory in a container has failed because the specified
|
|
// path does not refer to a directory.
|
|
var ErrExtractPointNotDirectory = errors.New("extraction point is not a directory")
|
|
|
|
// ContainerCopy performs a deprecated operation of archiving the resource at
|
|
// the specified path in the conatiner identified by the given name.
|
|
func (daemon *Daemon) ContainerCopy(name string, res string) (io.ReadCloser, error) {
|
|
container, err := daemon.Get(name)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
if res[0] == '/' || res[0] == '\\' {
|
|
res = res[1:]
|
|
}
|
|
|
|
return container.copy(res)
|
|
}
|
|
|
|
// ContainerStatPath stats the filesystem resource at the specified path in the
|
|
// container identified by the given name.
|
|
func (daemon *Daemon) ContainerStatPath(name string, path string) (stat *types.ContainerPathStat, err error) {
|
|
container, err := daemon.Get(name)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
return container.StatPath(path)
|
|
}
|
|
|
|
// ContainerArchivePath creates an archive of the filesystem resource at the
|
|
// specified path in the container identified by the given name. Returns a
|
|
// tar archive of the resource and whether it was a directory or a single file.
|
|
func (daemon *Daemon) ContainerArchivePath(name string, path string) (content io.ReadCloser, stat *types.ContainerPathStat, err error) {
|
|
container, err := daemon.Get(name)
|
|
if err != nil {
|
|
return nil, nil, err
|
|
}
|
|
|
|
return container.ArchivePath(path)
|
|
}
|
|
|
|
// ContainerExtractToDir extracts the given archive to the specified location
|
|
// in the filesystem of the container identified by the given name. The given
|
|
// path must be of a directory in the container. If it is not, the error will
|
|
// be ErrExtractPointNotDirectory. If noOverwriteDirNonDir is true then it will
|
|
// be an error if unpacking the given content would cause an existing directory
|
|
// to be replaced with a non-directory and vice versa.
|
|
func (daemon *Daemon) ContainerExtractToDir(name, path string, noOverwriteDirNonDir bool, content io.Reader) error {
|
|
container, err := daemon.Get(name)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
|
|
return container.ExtractToDir(path, noOverwriteDirNonDir, content)
|
|
}
|
|
|
|
// resolvePath resolves the given path in the container to a resource on the
|
|
// host. Returns a resolved path (absolute path to the resource on the host),
|
|
// the absolute path to the resource relative to the container's rootfs, and
|
|
// a error if the path points to outside the container's rootfs.
|
|
func (container *Container) resolvePath(path string) (resolvedPath, absPath string, err error) {
|
|
// Consider the given path as an absolute path in the container.
|
|
absPath = archive.PreserveTrailingDotOrSeparator(filepath.Join(string(filepath.Separator), path), path)
|
|
|
|
// Split the absPath into its Directory and Base components. We will
|
|
// resolve the dir in the scope of the container then append the base.
|
|
dirPath, basePath := filepath.Split(absPath)
|
|
|
|
resolvedDirPath, err := container.GetResourcePath(dirPath)
|
|
if err != nil {
|
|
return "", "", err
|
|
}
|
|
|
|
// resolvedDirPath will have been cleaned (no trailing path separators) so
|
|
// we can manually join it with the base path element.
|
|
resolvedPath = resolvedDirPath + string(filepath.Separator) + basePath
|
|
|
|
return resolvedPath, absPath, nil
|
|
}
|
|
|
|
// statPath is the unexported version of StatPath. Locks and mounts should
|
|
// be acquired before calling this method and the given path should be fully
|
|
// resolved to a path on the host corresponding to the given absolute path
|
|
// inside the container.
|
|
func (container *Container) statPath(resolvedPath, absPath string) (stat *types.ContainerPathStat, err error) {
|
|
lstat, err := os.Lstat(resolvedPath)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
var linkTarget string
|
|
if lstat.Mode()&os.ModeSymlink != 0 {
|
|
// Fully evaluate the symlink in the scope of the container rootfs.
|
|
hostPath, err := container.GetResourcePath(absPath)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
linkTarget, err = filepath.Rel(container.basefs, hostPath)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
// Make it an absolute path.
|
|
linkTarget = filepath.Join(string(filepath.Separator), linkTarget)
|
|
}
|
|
|
|
return &types.ContainerPathStat{
|
|
Name: filepath.Base(absPath),
|
|
Size: lstat.Size(),
|
|
Mode: lstat.Mode(),
|
|
Mtime: lstat.ModTime(),
|
|
LinkTarget: linkTarget,
|
|
}, nil
|
|
}
|
|
|
|
// StatPath stats the filesystem resource at the specified path in this
|
|
// container. Returns stat info about the resource.
|
|
func (container *Container) StatPath(path string) (stat *types.ContainerPathStat, err error) {
|
|
container.Lock()
|
|
defer container.Unlock()
|
|
|
|
if err = container.Mount(); err != nil {
|
|
return nil, err
|
|
}
|
|
defer container.Unmount()
|
|
|
|
err = container.mountVolumes()
|
|
defer container.unmountVolumes(true)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
resolvedPath, absPath, err := container.resolvePath(path)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
return container.statPath(resolvedPath, absPath)
|
|
}
|
|
|
|
// ArchivePath creates an archive of the filesystem resource at the specified
|
|
// path in this container. Returns a tar archive of the resource and stat info
|
|
// about the resource.
|
|
func (container *Container) ArchivePath(path string) (content io.ReadCloser, stat *types.ContainerPathStat, err error) {
|
|
container.Lock()
|
|
|
|
defer func() {
|
|
if err != nil {
|
|
// Wait to unlock the container until the archive is fully read
|
|
// (see the ReadCloseWrapper func below) or if there is an error
|
|
// before that occurs.
|
|
container.Unlock()
|
|
}
|
|
}()
|
|
|
|
if err = container.Mount(); err != nil {
|
|
return nil, nil, err
|
|
}
|
|
|
|
defer func() {
|
|
if err != nil {
|
|
// unmount any volumes
|
|
container.unmountVolumes(true)
|
|
// unmount the container's rootfs
|
|
container.Unmount()
|
|
}
|
|
}()
|
|
|
|
if err = container.mountVolumes(); err != nil {
|
|
return nil, nil, err
|
|
}
|
|
|
|
resolvedPath, absPath, err := container.resolvePath(path)
|
|
if err != nil {
|
|
return nil, nil, err
|
|
}
|
|
|
|
stat, err = container.statPath(resolvedPath, absPath)
|
|
if err != nil {
|
|
return nil, nil, err
|
|
}
|
|
|
|
// We need to rebase the archive entries if the last element of the
|
|
// resolved path was a symlink that was evaluated and is now different
|
|
// than the requested path. For example, if the given path was "/foo/bar/",
|
|
// but it resolved to "/var/lib/docker/containers/{id}/foo/baz/", we want
|
|
// to ensure that the archive entries start with "bar" and not "baz". This
|
|
// also catches the case when the root directory of the container is
|
|
// requested: we want the archive entries to start with "/" and not the
|
|
// container ID.
|
|
data, err := archive.TarResourceRebase(resolvedPath, filepath.Base(absPath))
|
|
if err != nil {
|
|
return nil, nil, err
|
|
}
|
|
|
|
content = ioutils.NewReadCloserWrapper(data, func() error {
|
|
err := data.Close()
|
|
container.unmountVolumes(true)
|
|
container.Unmount()
|
|
container.Unlock()
|
|
return err
|
|
})
|
|
|
|
container.logEvent("archive-path")
|
|
|
|
return content, stat, nil
|
|
}
|
|
|
|
// ExtractToDir extracts the given tar archive to the specified location in the
|
|
// filesystem of this container. The given path must be of a directory in the
|
|
// container. If it is not, the error will be ErrExtractPointNotDirectory. If
|
|
// noOverwriteDirNonDir is true then it will be an error if unpacking the
|
|
// given content would cause an existing directory to be replaced with a non-
|
|
// directory and vice versa.
|
|
func (container *Container) ExtractToDir(path string, noOverwriteDirNonDir bool, content io.Reader) (err error) {
|
|
container.Lock()
|
|
defer container.Unlock()
|
|
|
|
if err = container.Mount(); err != nil {
|
|
return err
|
|
}
|
|
defer container.Unmount()
|
|
|
|
err = container.mountVolumes()
|
|
defer container.unmountVolumes(true)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
|
|
// The destination path needs to be resolved to a host path, with all
|
|
// symbolic links followed in the scope of the container's rootfs. Note
|
|
// that we do not use `container.resolvePath(path)` here because we need
|
|
// to also evaluate the last path element if it is a symlink. This is so
|
|
// that you can extract an archive to a symlink that points to a directory.
|
|
|
|
// Consider the given path as an absolute path in the container.
|
|
absPath := archive.PreserveTrailingDotOrSeparator(filepath.Join(string(filepath.Separator), path), path)
|
|
|
|
// This will evaluate the last path element if it is a symlink.
|
|
resolvedPath, err := container.GetResourcePath(absPath)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
|
|
stat, err := os.Lstat(resolvedPath)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
|
|
if !stat.IsDir() {
|
|
return ErrExtractPointNotDirectory
|
|
}
|
|
|
|
// Need to check if the path is in a volume. If it is, it cannot be in a
|
|
// read-only volume. If it is not in a volume, the container cannot be
|
|
// configured with a read-only rootfs.
|
|
|
|
// Use the resolved path relative to the container rootfs as the new
|
|
// absPath. This way we fully follow any symlinks in a volume that may
|
|
// lead back outside the volume.
|
|
//
|
|
// The Windows implementation of filepath.Rel in golang 1.4 does not
|
|
// support volume style file path semantics. On Windows when using the
|
|
// filter driver, we are guaranteed that the path will always be
|
|
// a volume file path.
|
|
var baseRel string
|
|
if strings.HasPrefix(resolvedPath, `\\?\Volume{`) {
|
|
if strings.HasPrefix(resolvedPath, container.basefs) {
|
|
baseRel = resolvedPath[len(container.basefs):]
|
|
if baseRel[:1] == `\` {
|
|
baseRel = baseRel[1:]
|
|
}
|
|
}
|
|
} else {
|
|
baseRel, err = filepath.Rel(container.basefs, resolvedPath)
|
|
}
|
|
if err != nil {
|
|
return err
|
|
}
|
|
// Make it an absolute path.
|
|
absPath = filepath.Join(string(filepath.Separator), baseRel)
|
|
|
|
toVolume, err := checkIfPathIsInAVolume(container, absPath)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
|
|
if !toVolume && container.hostConfig.ReadonlyRootfs {
|
|
return ErrRootFSReadOnly
|
|
}
|
|
|
|
options := &archive.TarOptions{
|
|
ChownOpts: &archive.TarChownOptions{
|
|
UID: 0, GID: 0, // TODO: use config.User? Remap to userns root?
|
|
},
|
|
NoOverwriteDirNonDir: noOverwriteDirNonDir,
|
|
}
|
|
|
|
if err := chrootarchive.Untar(content, resolvedPath, options); err != nil {
|
|
return err
|
|
}
|
|
|
|
container.logEvent("extract-to-dir")
|
|
|
|
return nil
|
|
}
|