mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
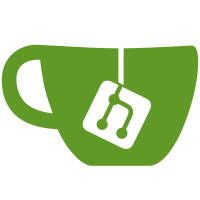
The BSD and Solaris versions of term.MakeRaw already set VMIN and VTIME explicitly such that a read returns when one character is available. cfmakeraw (which was previously used) in glibc also sets these values explicitly, so it should be done in the Linux version of MakeRaw as well to be consistent. Signed-off-by: Tobias Klauser <tklauser@distanz.ch>
39 lines
1 KiB
Go
39 lines
1 KiB
Go
package term
|
|
|
|
import (
|
|
"golang.org/x/sys/unix"
|
|
)
|
|
|
|
const (
|
|
getTermios = unix.TCGETS
|
|
setTermios = unix.TCSETS
|
|
)
|
|
|
|
// Termios is the Unix API for terminal I/O.
|
|
type Termios unix.Termios
|
|
|
|
// MakeRaw put the terminal connected to the given file descriptor into raw
|
|
// mode and returns the previous state of the terminal so that it can be
|
|
// restored.
|
|
func MakeRaw(fd uintptr) (*State, error) {
|
|
termios, err := unix.IoctlGetTermios(int(fd), getTermios)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
var oldState State
|
|
oldState.termios = Termios(*termios)
|
|
|
|
termios.Iflag &^= (unix.IGNBRK | unix.BRKINT | unix.PARMRK | unix.ISTRIP | unix.INLCR | unix.IGNCR | unix.ICRNL | unix.IXON)
|
|
termios.Oflag &^= unix.OPOST
|
|
termios.Lflag &^= (unix.ECHO | unix.ECHONL | unix.ICANON | unix.ISIG | unix.IEXTEN)
|
|
termios.Cflag &^= (unix.CSIZE | unix.PARENB)
|
|
termios.Cflag |= unix.CS8
|
|
termios.Cc[unix.VMIN] = 1
|
|
termios.Cc[unix.VTIME] = 0
|
|
|
|
if err := unix.IoctlSetTermios(int(fd), setTermios, termios); err != nil {
|
|
return nil, err
|
|
}
|
|
return &oldState, nil
|
|
}
|