mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
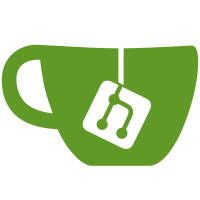
Signed-off-by: John Howard <jhoward@microsoft.com> If fixes an error in sameFsTime which was using `==` to compare two times. The correct way is to use go's built-in timea.Equals(timeb). In changes_windows, it uses sameFsTime to compare mTim of a `system.StatT` to allow TestChangesDirsMutated to operate correctly now. Note there is slight different between the Linux and Windows implementations of detecting changes. Due to https://github.com/moby/moby/issues/9874, and the fix at https://github.com/moby/moby/pull/11422, Linux does not consider a change to the directory time as a change. Windows on NTFS does. See https://github.com/moby/moby/pull/37982 for more information. The result in `TestChangesDirsMutated`, `dir3` is NOT considered a change in Linux, but IS considered a change on Windows. The test mutates dir3 to have a mtime of +1 second. With a handful of tests still outstanding, this change ports most of the unit tests under pkg/archive to Windows. It provides an implementation of `copyDir` in tests for Windows. To make a copy similar to Linux's `cp -a` while preserving timestamps and links to both valid and invalid targets, xcopy isn't sufficient. So I used robocopy, but had to circumvent certain exit codes that robocopy exits with which are warnings. Link to article describing this is in the code.
43 lines
1.3 KiB
Go
43 lines
1.3 KiB
Go
// +build !windows
|
|
|
|
package archive // import "github.com/docker/docker/pkg/archive"
|
|
|
|
import (
|
|
"os"
|
|
"syscall"
|
|
|
|
"github.com/docker/docker/pkg/system"
|
|
"golang.org/x/sys/unix"
|
|
)
|
|
|
|
func statDifferent(oldStat *system.StatT, newStat *system.StatT) bool {
|
|
// Don't look at size for dirs, its not a good measure of change
|
|
if oldStat.Mode() != newStat.Mode() ||
|
|
oldStat.UID() != newStat.UID() ||
|
|
oldStat.GID() != newStat.GID() ||
|
|
oldStat.Rdev() != newStat.Rdev() ||
|
|
// Don't look at size or modification time for dirs, its not a good
|
|
// measure of change. See https://github.com/moby/moby/issues/9874
|
|
// for a description of the issue with modification time, and
|
|
// https://github.com/moby/moby/pull/11422 for the change.
|
|
// (Note that in the Windows implementation of this function,
|
|
// modification time IS taken as a change). See
|
|
// https://github.com/moby/moby/pull/37982 for more information.
|
|
(oldStat.Mode()&unix.S_IFDIR != unix.S_IFDIR &&
|
|
(!sameFsTimeSpec(oldStat.Mtim(), newStat.Mtim()) || (oldStat.Size() != newStat.Size()))) {
|
|
return true
|
|
}
|
|
return false
|
|
}
|
|
|
|
func (info *FileInfo) isDir() bool {
|
|
return info.parent == nil || info.stat.Mode()&unix.S_IFDIR != 0
|
|
}
|
|
|
|
func getIno(fi os.FileInfo) uint64 {
|
|
return fi.Sys().(*syscall.Stat_t).Ino
|
|
}
|
|
|
|
func hasHardlinks(fi os.FileInfo) bool {
|
|
return fi.Sys().(*syscall.Stat_t).Nlink > 1
|
|
}
|