mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
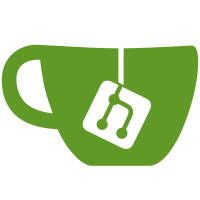
This fix tries to address the issue raised in 29975 where it was not possible to specify `attachable` flag for networks in compose format. NOTE: Compose format aleady supports `labels` in networks. This fix adds the support of `attachable` for compose v3.1 format. Additiona unit tests have been updated and added. This fix fixes 29975. Signed-off-by: Yong Tang <yong.tang.github@outlook.com>
134 lines
3 KiB
Go
134 lines
3 KiB
Go
package convert
|
|
|
|
import (
|
|
"testing"
|
|
|
|
"github.com/docker/docker/api/types"
|
|
"github.com/docker/docker/api/types/network"
|
|
composetypes "github.com/docker/docker/cli/compose/types"
|
|
"github.com/docker/docker/pkg/testutil/assert"
|
|
"github.com/docker/docker/pkg/testutil/tempfile"
|
|
)
|
|
|
|
func TestNamespaceScope(t *testing.T) {
|
|
scoped := Namespace{name: "foo"}.Scope("bar")
|
|
assert.Equal(t, scoped, "foo_bar")
|
|
}
|
|
|
|
func TestAddStackLabel(t *testing.T) {
|
|
labels := map[string]string{
|
|
"something": "labeled",
|
|
}
|
|
actual := AddStackLabel(Namespace{name: "foo"}, labels)
|
|
expected := map[string]string{
|
|
"something": "labeled",
|
|
LabelNamespace: "foo",
|
|
}
|
|
assert.DeepEqual(t, actual, expected)
|
|
}
|
|
|
|
func TestNetworks(t *testing.T) {
|
|
namespace := Namespace{name: "foo"}
|
|
serviceNetworks := map[string]struct{}{
|
|
"normal": {},
|
|
"outside": {},
|
|
"default": {},
|
|
"attachablenet": {},
|
|
}
|
|
source := networkMap{
|
|
"normal": composetypes.NetworkConfig{
|
|
Driver: "overlay",
|
|
DriverOpts: map[string]string{
|
|
"opt": "value",
|
|
},
|
|
Ipam: composetypes.IPAMConfig{
|
|
Driver: "driver",
|
|
Config: []*composetypes.IPAMPool{
|
|
{
|
|
Subnet: "10.0.0.0",
|
|
},
|
|
},
|
|
},
|
|
Labels: map[string]string{
|
|
"something": "labeled",
|
|
},
|
|
},
|
|
"outside": composetypes.NetworkConfig{
|
|
External: composetypes.External{
|
|
External: true,
|
|
Name: "special",
|
|
},
|
|
},
|
|
"attachablenet": composetypes.NetworkConfig{
|
|
Driver: "overlay",
|
|
Attachable: true,
|
|
},
|
|
}
|
|
expected := map[string]types.NetworkCreate{
|
|
"default": {
|
|
Labels: map[string]string{
|
|
LabelNamespace: "foo",
|
|
},
|
|
},
|
|
"normal": {
|
|
Driver: "overlay",
|
|
IPAM: &network.IPAM{
|
|
Driver: "driver",
|
|
Config: []network.IPAMConfig{
|
|
{
|
|
Subnet: "10.0.0.0",
|
|
},
|
|
},
|
|
},
|
|
Options: map[string]string{
|
|
"opt": "value",
|
|
},
|
|
Labels: map[string]string{
|
|
LabelNamespace: "foo",
|
|
"something": "labeled",
|
|
},
|
|
},
|
|
"attachablenet": {
|
|
Driver: "overlay",
|
|
Attachable: true,
|
|
Labels: map[string]string{
|
|
LabelNamespace: "foo",
|
|
},
|
|
},
|
|
}
|
|
|
|
networks, externals := Networks(namespace, source, serviceNetworks)
|
|
assert.DeepEqual(t, networks, expected)
|
|
assert.DeepEqual(t, externals, []string{"special"})
|
|
}
|
|
|
|
func TestSecrets(t *testing.T) {
|
|
namespace := Namespace{name: "foo"}
|
|
|
|
secretText := "this is the first secret"
|
|
secretFile := tempfile.NewTempFile(t, "convert-secrets", secretText)
|
|
defer secretFile.Remove()
|
|
|
|
source := map[string]composetypes.SecretConfig{
|
|
"one": {
|
|
File: secretFile.Name(),
|
|
Labels: map[string]string{"monster": "mash"},
|
|
},
|
|
"ext": {
|
|
External: composetypes.External{
|
|
External: true,
|
|
},
|
|
},
|
|
}
|
|
|
|
specs, err := Secrets(namespace, source)
|
|
assert.NilError(t, err)
|
|
assert.Equal(t, len(specs), 1)
|
|
secret := specs[0]
|
|
assert.Equal(t, secret.Name, "foo_one")
|
|
assert.DeepEqual(t, secret.Labels, map[string]string{
|
|
"monster": "mash",
|
|
LabelNamespace: "foo",
|
|
})
|
|
assert.DeepEqual(t, secret.Data, []byte(secretText))
|
|
}
|