mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
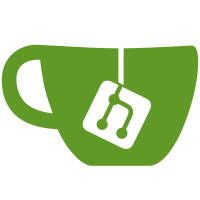
This adds support for Windows dockerd to run as a Windows service, managed by the service control manager. The log is written to the Windows event log (and can be viewed in the event viewer or in PowerShell). If there is a Go panic, the stack is written to a file panic.log in the Docker root. Signed-off-by: John Starks <jostarks@microsoft.com>
113 lines
2.9 KiB
Go
113 lines
2.9 KiB
Go
// +build !windows
|
|
|
|
package main
|
|
|
|
import (
|
|
"fmt"
|
|
"net"
|
|
"os"
|
|
"os/signal"
|
|
"path/filepath"
|
|
"strconv"
|
|
"syscall"
|
|
|
|
"github.com/docker/docker/daemon"
|
|
"github.com/docker/docker/libcontainerd"
|
|
"github.com/docker/docker/pkg/system"
|
|
"github.com/docker/libnetwork/portallocator"
|
|
)
|
|
|
|
const defaultDaemonConfigFile = "/etc/docker/daemon.json"
|
|
|
|
// currentUserIsOwner checks whether the current user is the owner of the given
|
|
// file.
|
|
func currentUserIsOwner(f string) bool {
|
|
if fileInfo, err := system.Stat(f); err == nil && fileInfo != nil {
|
|
if int(fileInfo.UID()) == os.Getuid() {
|
|
return true
|
|
}
|
|
}
|
|
return false
|
|
}
|
|
|
|
// setDefaultUmask sets the umask to 0022 to avoid problems
|
|
// caused by custom umask
|
|
func setDefaultUmask() error {
|
|
desiredUmask := 0022
|
|
syscall.Umask(desiredUmask)
|
|
if umask := syscall.Umask(desiredUmask); umask != desiredUmask {
|
|
return fmt.Errorf("failed to set umask: expected %#o, got %#o", desiredUmask, umask)
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
func getDaemonConfDir() string {
|
|
return "/etc/docker"
|
|
}
|
|
|
|
// setupConfigReloadTrap configures the USR2 signal to reload the configuration.
|
|
func (cli *DaemonCli) setupConfigReloadTrap() {
|
|
c := make(chan os.Signal, 1)
|
|
signal.Notify(c, syscall.SIGHUP)
|
|
go func() {
|
|
for range c {
|
|
cli.reloadConfig()
|
|
}
|
|
}()
|
|
}
|
|
|
|
func (cli *DaemonCli) getPlatformRemoteOptions() []libcontainerd.RemoteOption {
|
|
opts := []libcontainerd.RemoteOption{
|
|
libcontainerd.WithDebugLog(cli.Config.Debug),
|
|
}
|
|
if cli.Config.ContainerdAddr != "" {
|
|
opts = append(opts, libcontainerd.WithRemoteAddr(cli.Config.ContainerdAddr))
|
|
} else {
|
|
opts = append(opts, libcontainerd.WithStartDaemon(true))
|
|
}
|
|
if daemon.UsingSystemd(cli.Config) {
|
|
args := []string{"--systemd-cgroup=true"}
|
|
opts = append(opts, libcontainerd.WithRuntimeArgs(args))
|
|
}
|
|
return opts
|
|
}
|
|
|
|
// getLibcontainerdRoot gets the root directory for libcontainerd/containerd to
|
|
// store their state.
|
|
func (cli *DaemonCli) getLibcontainerdRoot() string {
|
|
return filepath.Join(cli.Config.ExecRoot, "libcontainerd")
|
|
}
|
|
|
|
// allocateDaemonPort ensures that there are no containers
|
|
// that try to use any port allocated for the docker server.
|
|
func allocateDaemonPort(addr string) error {
|
|
host, port, err := net.SplitHostPort(addr)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
|
|
intPort, err := strconv.Atoi(port)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
|
|
var hostIPs []net.IP
|
|
if parsedIP := net.ParseIP(host); parsedIP != nil {
|
|
hostIPs = append(hostIPs, parsedIP)
|
|
} else if hostIPs, err = net.LookupIP(host); err != nil {
|
|
return fmt.Errorf("failed to lookup %s address in host specification", host)
|
|
}
|
|
|
|
pa := portallocator.Get()
|
|
for _, hostIP := range hostIPs {
|
|
if _, err := pa.RequestPort(hostIP, "tcp", intPort); err != nil {
|
|
return fmt.Errorf("failed to allocate daemon listening port %d (err: %v)", intPort, err)
|
|
}
|
|
}
|
|
return nil
|
|
}
|
|
|
|
// notifyShutdown is called after the daemon shuts down but before the process exits.
|
|
func notifyShutdown(err error) {
|
|
}
|