mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
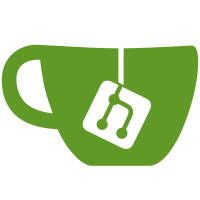
Signed-off-by: John Howard <jhoward@microsoft.com> This PR has the API changes described in https://github.com/moby/moby/issues/34617. Specifically, it adds an HTTP header "X-Requested-Platform" which is a JSON-encoded OCI Image-spec `Platform` structure. In addition, it renames (almost all) uses of a string variable platform (and associated) methods/functions to os. This makes it much clearer to disambiguate with the swarm "platform" which is really os/arch. This is a stepping stone to getting the daemon towards fully multi-platform/arch-aware, and makes it clear when "operating system" is being referred to rather than "platform" which is misleadingly used - sometimes in the swarm meaning, but more often as just the operating system.
94 lines
2.6 KiB
Go
94 lines
2.6 KiB
Go
package daemon
|
|
|
|
import (
|
|
"fmt"
|
|
"runtime"
|
|
|
|
"github.com/docker/distribution/reference"
|
|
"github.com/docker/docker/image"
|
|
"github.com/docker/docker/pkg/stringid"
|
|
)
|
|
|
|
// errImageDoesNotExist is error returned when no image can be found for a reference.
|
|
type errImageDoesNotExist struct {
|
|
ref reference.Reference
|
|
}
|
|
|
|
func (e errImageDoesNotExist) Error() string {
|
|
ref := e.ref
|
|
if named, ok := ref.(reference.Named); ok {
|
|
ref = reference.TagNameOnly(named)
|
|
}
|
|
return fmt.Sprintf("No such image: %s", reference.FamiliarString(ref))
|
|
}
|
|
|
|
func (e errImageDoesNotExist) NotFound() {}
|
|
|
|
// GetImageIDAndOS returns an image ID and operating system corresponding to the image referred to by
|
|
// refOrID.
|
|
func (daemon *Daemon) GetImageIDAndOS(refOrID string) (image.ID, string, error) {
|
|
ref, err := reference.ParseAnyReference(refOrID)
|
|
if err != nil {
|
|
return "", "", validationError{err}
|
|
}
|
|
namedRef, ok := ref.(reference.Named)
|
|
if !ok {
|
|
digested, ok := ref.(reference.Digested)
|
|
if !ok {
|
|
return "", "", errImageDoesNotExist{ref}
|
|
}
|
|
id := image.IDFromDigest(digested.Digest())
|
|
for platform := range daemon.stores {
|
|
if _, err = daemon.stores[platform].imageStore.Get(id); err == nil {
|
|
return id, platform, nil
|
|
}
|
|
}
|
|
return "", "", errImageDoesNotExist{ref}
|
|
}
|
|
|
|
if digest, err := daemon.referenceStore.Get(namedRef); err == nil {
|
|
// Search the image stores to get the operating system, defaulting to host OS.
|
|
imageOS := runtime.GOOS
|
|
id := image.IDFromDigest(digest)
|
|
for os := range daemon.stores {
|
|
if img, err := daemon.stores[os].imageStore.Get(id); err == nil {
|
|
imageOS = img.OperatingSystem()
|
|
break
|
|
}
|
|
}
|
|
return id, imageOS, nil
|
|
}
|
|
|
|
// deprecated: repo:shortid https://github.com/docker/docker/pull/799
|
|
if tagged, ok := namedRef.(reference.Tagged); ok {
|
|
if tag := tagged.Tag(); stringid.IsShortID(stringid.TruncateID(tag)) {
|
|
for platform := range daemon.stores {
|
|
if id, err := daemon.stores[platform].imageStore.Search(tag); err == nil {
|
|
for _, storeRef := range daemon.referenceStore.References(id.Digest()) {
|
|
if storeRef.Name() == namedRef.Name() {
|
|
return id, platform, nil
|
|
}
|
|
}
|
|
}
|
|
}
|
|
}
|
|
}
|
|
|
|
// Search based on ID
|
|
for os := range daemon.stores {
|
|
if id, err := daemon.stores[os].imageStore.Search(refOrID); err == nil {
|
|
return id, os, nil
|
|
}
|
|
}
|
|
|
|
return "", "", errImageDoesNotExist{ref}
|
|
}
|
|
|
|
// GetImage returns an image corresponding to the image referred to by refOrID.
|
|
func (daemon *Daemon) GetImage(refOrID string) (*image.Image, error) {
|
|
imgID, os, err := daemon.GetImageIDAndOS(refOrID)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return daemon.stores[os].imageStore.Get(imgID)
|
|
}
|