mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
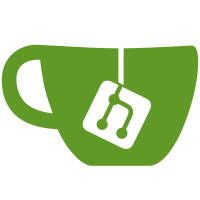
Use strongly typed errors to set HTTP status codes. Error interfaces are defined in the api/errors package and errors returned from controllers are checked against these interfaces. Errors can be wraeped in a pkg/errors.Causer, as long as somewhere in the line of causes one of the interfaces is implemented. The special error interfaces take precedence over Causer, meaning if both Causer and one of the new error interfaces are implemented, the Causer is not traversed. Signed-off-by: Brian Goff <cpuguy83@gmail.com>
57 lines
1.5 KiB
Go
57 lines
1.5 KiB
Go
// +build !windows
|
|
|
|
package daemon
|
|
|
|
import (
|
|
"github.com/docker/docker/api/types"
|
|
"github.com/docker/docker/container"
|
|
"github.com/pkg/errors"
|
|
)
|
|
|
|
// Resolve Network SandboxID in case the container reuse another container's network stack
|
|
func (daemon *Daemon) getNetworkSandboxID(c *container.Container) (string, error) {
|
|
curr := c
|
|
for curr.HostConfig.NetworkMode.IsContainer() {
|
|
containerID := curr.HostConfig.NetworkMode.ConnectedContainer()
|
|
connected, err := daemon.GetContainer(containerID)
|
|
if err != nil {
|
|
return "", errors.Wrapf(err, "Could not get container for %s", containerID)
|
|
}
|
|
curr = connected
|
|
}
|
|
return curr.NetworkSettings.SandboxID, nil
|
|
}
|
|
|
|
func (daemon *Daemon) getNetworkStats(c *container.Container) (map[string]types.NetworkStats, error) {
|
|
sandboxID, err := daemon.getNetworkSandboxID(c)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
sb, err := daemon.netController.SandboxByID(sandboxID)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
lnstats, err := sb.Statistics()
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
stats := make(map[string]types.NetworkStats)
|
|
// Convert libnetwork nw stats into api stats
|
|
for ifName, ifStats := range lnstats {
|
|
stats[ifName] = types.NetworkStats{
|
|
RxBytes: ifStats.RxBytes,
|
|
RxPackets: ifStats.RxPackets,
|
|
RxErrors: ifStats.RxErrors,
|
|
RxDropped: ifStats.RxDropped,
|
|
TxBytes: ifStats.TxBytes,
|
|
TxPackets: ifStats.TxPackets,
|
|
TxErrors: ifStats.TxErrors,
|
|
TxDropped: ifStats.TxDropped,
|
|
}
|
|
}
|
|
|
|
return stats, nil
|
|
}
|