mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
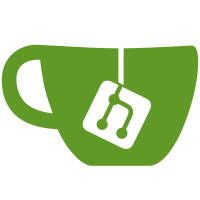
lxc is special in that we cannot create the master outside of the container without opening the slave because we have nothing to provide to the cmd. We have to open both then do the crazy setup on command right now instead of passing the console path to lxc and telling it to open up that console. we save a couple of openfiles in the native driver because we can do this. Docker-DCO-1.1-Signed-off-by: Michael Crosby <michael@docker.com> (github: crosbymichael)
46 lines
781 B
Go
46 lines
781 B
Go
package execdriver
|
|
|
|
import (
|
|
"io"
|
|
"os/exec"
|
|
)
|
|
|
|
type StdConsole struct {
|
|
}
|
|
|
|
func NewStdConsole(command *Command, pipes *Pipes) (*StdConsole, error) {
|
|
std := &StdConsole{}
|
|
|
|
if err := std.AttachPipes(&command.Cmd, pipes); err != nil {
|
|
return nil, err
|
|
}
|
|
return std, nil
|
|
}
|
|
|
|
func (s *StdConsole) AttachPipes(command *exec.Cmd, pipes *Pipes) error {
|
|
command.Stdout = pipes.Stdout
|
|
command.Stderr = pipes.Stderr
|
|
|
|
if pipes.Stdin != nil {
|
|
stdin, err := command.StdinPipe()
|
|
if err != nil {
|
|
return err
|
|
}
|
|
|
|
go func() {
|
|
defer stdin.Close()
|
|
io.Copy(stdin, pipes.Stdin)
|
|
}()
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (s *StdConsole) Resize(h, w int) error {
|
|
// we do not need to reside a non tty
|
|
return nil
|
|
}
|
|
|
|
func (s *StdConsole) Close() error {
|
|
// nothing to close here
|
|
return nil
|
|
}
|