mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
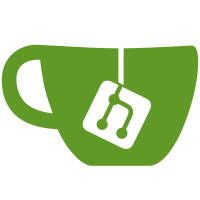
It will Tar up contents of child directory onto tmpfs if mounted over This patch will use the new PreMount and PostMount hooks to "tar" up the contents of the base image on top of tmpfs mount points. Signed-off-by: Dan Walsh <dwalsh@redhat.com>
56 lines
1.5 KiB
Go
56 lines
1.5 KiB
Go
package native
|
|
|
|
import (
|
|
"fmt"
|
|
"os"
|
|
"os/exec"
|
|
"strings"
|
|
|
|
"github.com/Sirupsen/logrus"
|
|
"github.com/opencontainers/runc/libcontainer/configs"
|
|
)
|
|
|
|
func genTmpfsPremountCmd(tmpDir string, fullDest string, dest string) []configs.Command {
|
|
var premount []configs.Command
|
|
tarPath, err := exec.LookPath("tar")
|
|
if err != nil {
|
|
logrus.Warn("tar command is not available for tmpfs mount: %s", err)
|
|
return premount
|
|
}
|
|
if _, err = exec.LookPath("rm"); err != nil {
|
|
logrus.Warn("rm command is not available for tmpfs mount: %s", err)
|
|
return premount
|
|
}
|
|
tarFile := fmt.Sprintf("%s/%s.tar", tmpDir, strings.Replace(dest, "/", "_", -1))
|
|
if _, err := os.Stat(fullDest); err == nil {
|
|
premount = append(premount, configs.Command{
|
|
Path: tarPath,
|
|
Args: []string{"-cf", tarFile, "-C", fullDest, "."},
|
|
})
|
|
}
|
|
return premount
|
|
}
|
|
|
|
func genTmpfsPostmountCmd(tmpDir string, fullDest string, dest string) []configs.Command {
|
|
var postmount []configs.Command
|
|
tarPath, err := exec.LookPath("tar")
|
|
if err != nil {
|
|
return postmount
|
|
}
|
|
rmPath, err := exec.LookPath("rm")
|
|
if err != nil {
|
|
return postmount
|
|
}
|
|
if _, err := os.Stat(fullDest); os.IsNotExist(err) {
|
|
return postmount
|
|
}
|
|
tarFile := fmt.Sprintf("%s/%s.tar", tmpDir, strings.Replace(dest, "/", "_", -1))
|
|
postmount = append(postmount, configs.Command{
|
|
Path: tarPath,
|
|
Args: []string{"-xf", tarFile, "-C", fullDest, "."},
|
|
})
|
|
return append(postmount, configs.Command{
|
|
Path: rmPath,
|
|
Args: []string{"-f", tarFile},
|
|
})
|
|
}
|