mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
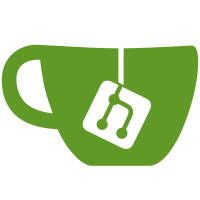
Before this, if `forceRemove` is set the container data will be removed no matter what, including if there are issues with removing container on-disk state (rw layer, container root). In practice this causes a lot of issues with leaked data sitting on disk that users are not able to clean up themselves. This is particularly a problem while the `EBUSY` errors on remove are so prevalent. So for now let's not keep this behavior. Signed-off-by: Brian Goff <cpuguy83@gmail.com>
54 lines
1.3 KiB
Go
54 lines
1.3 KiB
Go
package mount
|
|
|
|
// Info reveals information about a particular mounted filesystem. This
|
|
// struct is populated from the content in the /proc/<pid>/mountinfo file.
|
|
type Info struct {
|
|
// ID is a unique identifier of the mount (may be reused after umount).
|
|
ID int
|
|
|
|
// Parent indicates the ID of the mount parent (or of self for the top of the
|
|
// mount tree).
|
|
Parent int
|
|
|
|
// Major indicates one half of the device ID which identifies the device class.
|
|
Major int
|
|
|
|
// Minor indicates one half of the device ID which identifies a specific
|
|
// instance of device.
|
|
Minor int
|
|
|
|
// Root of the mount within the filesystem.
|
|
Root string
|
|
|
|
// Mountpoint indicates the mount point relative to the process's root.
|
|
Mountpoint string
|
|
|
|
// Opts represents mount-specific options.
|
|
Opts string
|
|
|
|
// Optional represents optional fields.
|
|
Optional string
|
|
|
|
// Fstype indicates the type of filesystem, such as EXT3.
|
|
Fstype string
|
|
|
|
// Source indicates filesystem specific information or "none".
|
|
Source string
|
|
|
|
// VfsOpts represents per super block options.
|
|
VfsOpts string
|
|
}
|
|
|
|
type byMountpoint []*Info
|
|
|
|
func (by byMountpoint) Len() int {
|
|
return len(by)
|
|
}
|
|
|
|
func (by byMountpoint) Less(i, j int) bool {
|
|
return by[i].Mountpoint < by[j].Mountpoint
|
|
}
|
|
|
|
func (by byMountpoint) Swap(i, j int) {
|
|
by[i], by[j] = by[j], by[i]
|
|
}
|