mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
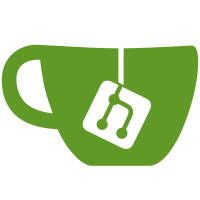
Turn BytesPipe's Read and Write functions into blocking, goroutine-safe functions. Add a CloseWithError function to propagate an error code to the Read function. Adjust tests to work with the blocking Read and Write functions. Remove BufReader, since now its users can use BytesPipe directly. Signed-off-by: Aaron Lehmann <aaron.lehmann@docker.com>
67 lines
1.8 KiB
Go
67 lines
1.8 KiB
Go
package ioutils
|
|
|
|
import (
|
|
"fmt"
|
|
"strings"
|
|
"testing"
|
|
)
|
|
|
|
// Implement io.Reader
|
|
type errorReader struct{}
|
|
|
|
func (r *errorReader) Read(p []byte) (int, error) {
|
|
return 0, fmt.Errorf("Error reader always fail.")
|
|
}
|
|
|
|
func TestReadCloserWrapperClose(t *testing.T) {
|
|
reader := strings.NewReader("A string reader")
|
|
wrapper := NewReadCloserWrapper(reader, func() error {
|
|
return fmt.Errorf("This will be called when closing")
|
|
})
|
|
err := wrapper.Close()
|
|
if err == nil || !strings.Contains(err.Error(), "This will be called when closing") {
|
|
t.Fatalf("readCloserWrapper should have call the anonymous func and thus, fail.")
|
|
}
|
|
}
|
|
|
|
func TestReaderErrWrapperReadOnError(t *testing.T) {
|
|
called := false
|
|
reader := &errorReader{}
|
|
wrapper := NewReaderErrWrapper(reader, func() {
|
|
called = true
|
|
})
|
|
_, err := wrapper.Read([]byte{})
|
|
if err == nil || !strings.Contains(err.Error(), "Error reader always fail.") {
|
|
t.Fatalf("readErrWrapper should returned an error")
|
|
}
|
|
if !called {
|
|
t.Fatalf("readErrWrapper should have call the anonymous function on failure")
|
|
}
|
|
}
|
|
|
|
func TestReaderErrWrapperRead(t *testing.T) {
|
|
reader := strings.NewReader("a string reader.")
|
|
wrapper := NewReaderErrWrapper(reader, func() {
|
|
t.Fatalf("readErrWrapper should not have called the anonymous function")
|
|
})
|
|
// Read 20 byte (should be ok with the string above)
|
|
num, err := wrapper.Read(make([]byte, 20))
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
if num != 16 {
|
|
t.Fatalf("readerErrWrapper should have read 16 byte, but read %d", num)
|
|
}
|
|
}
|
|
|
|
func TestHashData(t *testing.T) {
|
|
reader := strings.NewReader("hash-me")
|
|
actual, err := HashData(reader)
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
expected := "sha256:4d11186aed035cc624d553e10db358492c84a7cd6b9670d92123c144930450aa"
|
|
if actual != expected {
|
|
t.Fatalf("Expecting %s, got %s", expected, actual)
|
|
}
|
|
}
|