mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
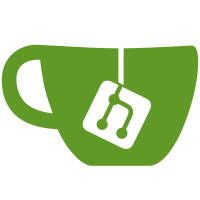
- Refactor opts.ValidatePath and add an opts.ValidateDevice ValidePath will now accept : containerPath:mode, hostPath:containerPath:mode and hostPath:containerPath. ValidateDevice will have the same behavior as current. - Refactor opts.ValidateEnv, opts.ParseEnvFile Environment variables will now be validated with the following definition : > Environment variables set by the user must have a name consisting > solely of alphabetics, numerics, and underscores - the first of > which must not be numeric. Signed-off-by: Vincent Demeester <vincent@sbr.pm>
35 lines
471 B
Go
35 lines
471 B
Go
package opts
|
|
|
|
import (
|
|
"fmt"
|
|
"net"
|
|
)
|
|
|
|
// IpOpt type that hold an IP
|
|
type IpOpt struct {
|
|
*net.IP
|
|
}
|
|
|
|
func NewIpOpt(ref *net.IP, defaultVal string) *IpOpt {
|
|
o := &IpOpt{
|
|
IP: ref,
|
|
}
|
|
o.Set(defaultVal)
|
|
return o
|
|
}
|
|
|
|
func (o *IpOpt) Set(val string) error {
|
|
ip := net.ParseIP(val)
|
|
if ip == nil {
|
|
return fmt.Errorf("%s is not an ip address", val)
|
|
}
|
|
*o.IP = ip
|
|
return nil
|
|
}
|
|
|
|
func (o *IpOpt) String() string {
|
|
if *o.IP == nil {
|
|
return ""
|
|
}
|
|
return o.IP.String()
|
|
}
|