mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
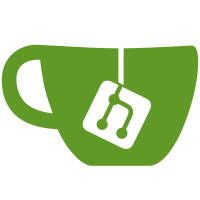
The containerd client is very chatty at the best of times. Because the libcontained API is stateless and references containers and processes by string ID for every method call, the implementation is essentially forced to use the containerd client in a way which amplifies the number of redundant RPCs invoked to perform any operation. The libcontainerd remote implementation has to reload the containerd container, task and/or process metadata for nearly every operation. This in turn amplifies the number of context switches between dockerd and containerd to perform any container operation or handle a containerd event, increasing the load on the system which could otherwise be allocated to workloads. Overhaul the libcontainerd interface to reduce the impedance mismatch with the containerd client so that the containerd client can be used more efficiently. Split the API out into container, task and process interfaces which the consumer is expected to retain so that libcontainerd can retain state---especially the analogous containerd client objects---without having to manage any state-store inside the libcontainerd client. Signed-off-by: Cory Snider <csnider@mirantis.com>
100 lines
2.5 KiB
Go
100 lines
2.5 KiB
Go
//go:build linux
|
|
// +build linux
|
|
|
|
package daemon
|
|
|
|
import (
|
|
"context"
|
|
"testing"
|
|
|
|
"github.com/docker/docker/container"
|
|
"github.com/docker/docker/libcontainerd/types"
|
|
"gotest.tools/v3/assert"
|
|
)
|
|
|
|
// This test simply verify that when a wrong ID used, a specific error should be returned for exec resize.
|
|
func TestExecResizeNoSuchExec(t *testing.T) {
|
|
n := "TestExecResize"
|
|
d := &Daemon{
|
|
execCommands: container.NewExecStore(),
|
|
}
|
|
c := &container.Container{
|
|
ExecCommands: container.NewExecStore(),
|
|
}
|
|
ec := &container.ExecConfig{
|
|
ID: n,
|
|
Container: c,
|
|
}
|
|
d.registerExecCommand(c, ec)
|
|
err := d.ContainerExecResize("nil", 24, 8)
|
|
assert.ErrorContains(t, err, "No such exec instance")
|
|
}
|
|
|
|
type execResizeMockProcess struct {
|
|
types.Process
|
|
Width, Height int
|
|
}
|
|
|
|
func (p *execResizeMockProcess) Resize(ctx context.Context, width, height uint32) error {
|
|
p.Width = int(width)
|
|
p.Height = int(height)
|
|
return nil
|
|
}
|
|
|
|
// This test is to make sure that when exec context is ready, resize should call ResizeTerminal via containerd client.
|
|
func TestExecResize(t *testing.T) {
|
|
n := "TestExecResize"
|
|
width := 24
|
|
height := 8
|
|
mp := &execResizeMockProcess{}
|
|
d := &Daemon{
|
|
execCommands: container.NewExecStore(),
|
|
containers: container.NewMemoryStore(),
|
|
}
|
|
c := &container.Container{
|
|
ID: n,
|
|
ExecCommands: container.NewExecStore(),
|
|
State: &container.State{Running: true},
|
|
}
|
|
ec := &container.ExecConfig{
|
|
ID: n,
|
|
Container: c,
|
|
Process: mp,
|
|
Started: make(chan struct{}),
|
|
}
|
|
close(ec.Started)
|
|
d.containers.Add(n, c)
|
|
d.registerExecCommand(c, ec)
|
|
err := d.ContainerExecResize(n, height, width)
|
|
assert.NilError(t, err)
|
|
assert.Equal(t, mp.Width, width)
|
|
assert.Equal(t, mp.Height, height)
|
|
}
|
|
|
|
// This test is to make sure that when exec context is not ready, a timeout error should happen.
|
|
// TODO: the expect running time for this test is 10s, which would be too long for unit test.
|
|
func TestExecResizeTimeout(t *testing.T) {
|
|
n := "TestExecResize"
|
|
width := 24
|
|
height := 8
|
|
mp := &execResizeMockProcess{}
|
|
d := &Daemon{
|
|
execCommands: container.NewExecStore(),
|
|
containers: container.NewMemoryStore(),
|
|
}
|
|
c := &container.Container{
|
|
ID: n,
|
|
ExecCommands: container.NewExecStore(),
|
|
State: &container.State{Running: true},
|
|
}
|
|
ec := &container.ExecConfig{
|
|
ID: n,
|
|
Container: c,
|
|
Process: mp,
|
|
Started: make(chan struct{}),
|
|
}
|
|
d.containers.Add(n, c)
|
|
d.registerExecCommand(c, ec)
|
|
err := d.ContainerExecResize(n, height, width)
|
|
assert.ErrorContains(t, err, "timeout waiting for exec session ready")
|
|
}
|