mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
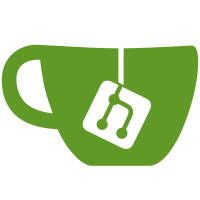
update bash commpletion for pids limit update check config for kernel add docs for pids limit add pids stats add stats to docker client Signed-off-by: Jessica Frazelle <acidburn@docker.com>
106 lines
2.6 KiB
Go
106 lines
2.6 KiB
Go
// +build !windows
|
|
|
|
package main
|
|
|
|
import (
|
|
"github.com/docker/docker/pkg/sysinfo"
|
|
)
|
|
|
|
var (
|
|
// SysInfo stores information about which features a kernel supports.
|
|
SysInfo *sysinfo.SysInfo
|
|
cpuCfsPeriod = testRequirement{
|
|
func() bool {
|
|
return SysInfo.CPUCfsPeriod
|
|
},
|
|
"Test requires an environment that supports cgroup cfs period.",
|
|
}
|
|
cpuCfsQuota = testRequirement{
|
|
func() bool {
|
|
return SysInfo.CPUCfsQuota
|
|
},
|
|
"Test requires an environment that supports cgroup cfs quota.",
|
|
}
|
|
cpuShare = testRequirement{
|
|
func() bool {
|
|
return SysInfo.CPUShares
|
|
},
|
|
"Test requires an environment that supports cgroup cpu shares.",
|
|
}
|
|
oomControl = testRequirement{
|
|
func() bool {
|
|
return SysInfo.OomKillDisable
|
|
},
|
|
"Test requires Oom control enabled.",
|
|
}
|
|
pidsLimit = testRequirement{
|
|
func() bool {
|
|
return SysInfo.PidsLimit
|
|
},
|
|
"Test requires pids limit enabled.",
|
|
}
|
|
kernelMemorySupport = testRequirement{
|
|
func() bool {
|
|
return SysInfo.KernelMemory
|
|
},
|
|
"Test requires an environment that supports cgroup kernel memory.",
|
|
}
|
|
memoryLimitSupport = testRequirement{
|
|
func() bool {
|
|
return SysInfo.MemoryLimit
|
|
},
|
|
"Test requires an environment that supports cgroup memory limit.",
|
|
}
|
|
memoryReservationSupport = testRequirement{
|
|
func() bool {
|
|
return SysInfo.MemoryReservation
|
|
},
|
|
"Test requires an environment that supports cgroup memory reservation.",
|
|
}
|
|
swapMemorySupport = testRequirement{
|
|
func() bool {
|
|
return SysInfo.SwapLimit
|
|
},
|
|
"Test requires an environment that supports cgroup swap memory limit.",
|
|
}
|
|
memorySwappinessSupport = testRequirement{
|
|
func() bool {
|
|
return SysInfo.MemorySwappiness
|
|
},
|
|
"Test requires an environment that supports cgroup memory swappiness.",
|
|
}
|
|
blkioWeight = testRequirement{
|
|
func() bool {
|
|
return SysInfo.BlkioWeight
|
|
},
|
|
"Test requires an environment that supports blkio weight.",
|
|
}
|
|
cgroupCpuset = testRequirement{
|
|
func() bool {
|
|
return SysInfo.Cpuset
|
|
},
|
|
"Test requires an environment that supports cgroup cpuset.",
|
|
}
|
|
seccompEnabled = testRequirement{
|
|
func() bool {
|
|
return supportsSeccomp && SysInfo.Seccomp
|
|
},
|
|
"Test requires that seccomp support be enabled in the daemon.",
|
|
}
|
|
bridgeNfIptables = testRequirement{
|
|
func() bool {
|
|
return !SysInfo.BridgeNFCallIPTablesDisabled
|
|
},
|
|
"Test requires that bridge-nf-call-iptables support be enabled in the daemon.",
|
|
}
|
|
bridgeNfIP6tables = testRequirement{
|
|
func() bool {
|
|
return !SysInfo.BridgeNFCallIP6TablesDisabled
|
|
},
|
|
"Test requires that bridge-nf-call-ip6tables support be enabled in the daemon.",
|
|
}
|
|
)
|
|
|
|
func init() {
|
|
SysInfo = sysinfo.New(true)
|
|
}
|