mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
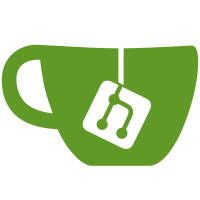
summary of changes: registry/auth.go - More logging around the login functions - split Login() out to handle different code paths for v1 (unchanged logic) and v2 (does not currently do account creation) - handling for either basic or token based login attempts registry/authchallenge.go - New File - credit to Brian Bland <brian.bland@docker.com> (github: BrianBland) - handles parsing of WWW-Authenticate response headers registry/endpoint.go - EVEN MOAR LOGGING - Many edits throught to make the coad less dense. Sparse code is more readable code. - slit Ping() out to handle different code paths for v1 (unchanged logic) and v2. - Updated Endpoint struct type to include an entry for authorization challenges discovered during ping of a v2 registry. - If registry endpoint version is unknown, v2 code path is first attempted, then fallback to v1 upon failure. registry/service.go - STILL MOAR LOGGING - simplified the logic around starting the 'auth' job. registry/session.go - updated use of a registry.Endpoint struct field. registry/token.go - New File - Handles getting token from the parameters of a token auth challenge. - Modified from function written by Brian Bland (see above credit). registry/types.go - Removed 'DefaultAPIVersion' in lieu of 'APIVersionUnknown = 0'` Docker-DCO-1.1-Signed-off-by: Josh Hawn <josh.hawn@docker.com> (github: jlhawn)
109 lines
2.4 KiB
Go
109 lines
2.4 KiB
Go
package registry
|
|
|
|
type SearchResult struct {
|
|
StarCount int `json:"star_count"`
|
|
IsOfficial bool `json:"is_official"`
|
|
Name string `json:"name"`
|
|
IsTrusted bool `json:"is_trusted"`
|
|
Description string `json:"description"`
|
|
}
|
|
|
|
type SearchResults struct {
|
|
Query string `json:"query"`
|
|
NumResults int `json:"num_results"`
|
|
Results []SearchResult `json:"results"`
|
|
}
|
|
|
|
type RepositoryData struct {
|
|
ImgList map[string]*ImgData
|
|
Endpoints []string
|
|
Tokens []string
|
|
}
|
|
|
|
type ImgData struct {
|
|
ID string `json:"id"`
|
|
Checksum string `json:"checksum,omitempty"`
|
|
ChecksumPayload string `json:"-"`
|
|
Tag string `json:",omitempty"`
|
|
}
|
|
|
|
type RegistryInfo struct {
|
|
Version string `json:"version"`
|
|
Standalone bool `json:"standalone"`
|
|
}
|
|
|
|
type FSLayer struct {
|
|
BlobSum string `json:"blobSum"`
|
|
}
|
|
|
|
type ManifestHistory struct {
|
|
V1Compatibility string `json:"v1Compatibility"`
|
|
}
|
|
|
|
type ManifestData struct {
|
|
Name string `json:"name"`
|
|
Tag string `json:"tag"`
|
|
Architecture string `json:"architecture"`
|
|
FSLayers []*FSLayer `json:"fsLayers"`
|
|
History []*ManifestHistory `json:"history"`
|
|
SchemaVersion int `json:"schemaVersion"`
|
|
}
|
|
|
|
type APIVersion int
|
|
|
|
func (av APIVersion) String() string {
|
|
return apiVersions[av]
|
|
}
|
|
|
|
var apiVersions = map[APIVersion]string{
|
|
1: "v1",
|
|
2: "v2",
|
|
}
|
|
|
|
// API Version identifiers.
|
|
const (
|
|
APIVersionUnknown = iota
|
|
APIVersion1
|
|
APIVersion2
|
|
)
|
|
|
|
// RepositoryInfo Examples:
|
|
// {
|
|
// "Index" : {
|
|
// "Name" : "docker.io",
|
|
// "Mirrors" : ["https://registry-2.docker.io/v1/", "https://registry-3.docker.io/v1/"],
|
|
// "Secure" : true,
|
|
// "Official" : true,
|
|
// },
|
|
// "RemoteName" : "library/debian",
|
|
// "LocalName" : "debian",
|
|
// "CanonicalName" : "docker.io/debian"
|
|
// "Official" : true,
|
|
// }
|
|
|
|
// {
|
|
// "Index" : {
|
|
// "Name" : "127.0.0.1:5000",
|
|
// "Mirrors" : [],
|
|
// "Secure" : false,
|
|
// "Official" : false,
|
|
// },
|
|
// "RemoteName" : "user/repo",
|
|
// "LocalName" : "127.0.0.1:5000/user/repo",
|
|
// "CanonicalName" : "127.0.0.1:5000/user/repo",
|
|
// "Official" : false,
|
|
// }
|
|
type IndexInfo struct {
|
|
Name string
|
|
Mirrors []string
|
|
Secure bool
|
|
Official bool
|
|
}
|
|
|
|
type RepositoryInfo struct {
|
|
Index *IndexInfo
|
|
RemoteName string
|
|
LocalName string
|
|
CanonicalName string
|
|
Official bool
|
|
}
|