mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
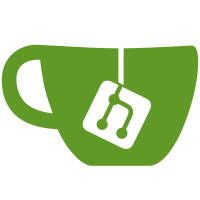
Although having a request ID available throughout the codebase is very valuable, the impact of requiring a Context as an argument to every function in the codepath of an API request, is too significant and was not properly understood at the time of the review. Furthermore, mixing API-layer code with non-API-layer code makes the latter usable only by API-layer code (one that has a notion of Context). This reverts commitde41640435
, reversing changes made to7daeecd42d
. Signed-off-by: Tibor Vass <tibor@docker.com> Conflicts: api/server/container.go builder/internals.go daemon/container_unix.go daemon/create.go
64 lines
1.7 KiB
Go
64 lines
1.7 KiB
Go
package daemon
|
|
|
|
import (
|
|
"io"
|
|
|
|
"github.com/docker/docker/pkg/stdcopy"
|
|
)
|
|
|
|
// ContainerAttachWithLogsConfig holds the streams to use when connecting to a container to view logs.
|
|
type ContainerAttachWithLogsConfig struct {
|
|
InStream io.ReadCloser
|
|
OutStream io.Writer
|
|
UseStdin, UseStdout, UseStderr bool
|
|
Logs, Stream bool
|
|
}
|
|
|
|
// ContainerAttachWithLogs attaches to logs according to the config passed in. See ContainerAttachWithLogsConfig.
|
|
func (daemon *Daemon) ContainerAttachWithLogs(prefixOrName string, c *ContainerAttachWithLogsConfig) error {
|
|
container, err := daemon.Get(prefixOrName)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
|
|
var errStream io.Writer
|
|
|
|
if !container.Config.Tty {
|
|
errStream = stdcopy.NewStdWriter(c.OutStream, stdcopy.Stderr)
|
|
c.OutStream = stdcopy.NewStdWriter(c.OutStream, stdcopy.Stdout)
|
|
} else {
|
|
errStream = c.OutStream
|
|
}
|
|
|
|
var stdin io.ReadCloser
|
|
var stdout, stderr io.Writer
|
|
|
|
if c.UseStdin {
|
|
stdin = c.InStream
|
|
}
|
|
if c.UseStdout {
|
|
stdout = c.OutStream
|
|
}
|
|
if c.UseStderr {
|
|
stderr = errStream
|
|
}
|
|
|
|
return container.attachWithLogs(stdin, stdout, stderr, c.Logs, c.Stream)
|
|
}
|
|
|
|
// ContainerWsAttachWithLogsConfig attach with websockets, since all
|
|
// stream data is delegated to the websocket to handle there.
|
|
type ContainerWsAttachWithLogsConfig struct {
|
|
InStream io.ReadCloser
|
|
OutStream, ErrStream io.Writer
|
|
Logs, Stream bool
|
|
}
|
|
|
|
// ContainerWsAttachWithLogs websocket connection
|
|
func (daemon *Daemon) ContainerWsAttachWithLogs(prefixOrName string, c *ContainerWsAttachWithLogsConfig) error {
|
|
container, err := daemon.Get(prefixOrName)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
return container.attachWithLogs(c.InStream, c.OutStream, c.ErrStream, c.Logs, c.Stream)
|
|
}
|