mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
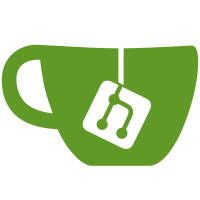
Manifest is now generated during a v2 push, not relying on previously generated hashes. When pushing a layer, the hash is directly calculated from the tar contents which will be pushed. Computing the hash on push ensures that the hash contents always match what is seen by the registry. This also mitigates issues with tarsum differences and permits using pure SHA digests. Additionally the new manifest function is moved to the unit tests since it is no longer called outside the tests. Signed-off-by: Derek McGowan <derek@mcgstyle.net> (github: dmcgowan)
158 lines
5.4 KiB
Go
158 lines
5.4 KiB
Go
package main
|
|
|
|
import (
|
|
"fmt"
|
|
"io/ioutil"
|
|
"os"
|
|
"os/exec"
|
|
"strings"
|
|
"testing"
|
|
"time"
|
|
|
|
"github.com/docker/docker/vendor/src/code.google.com/p/go/src/pkg/archive/tar"
|
|
)
|
|
|
|
// pulling an image from the central registry should work
|
|
func TestPushBusyboxImage(t *testing.T) {
|
|
defer setupRegistry(t)()
|
|
|
|
repoName := fmt.Sprintf("%v/dockercli/busybox", privateRegistryURL)
|
|
// tag the image to upload it tot he private registry
|
|
tagCmd := exec.Command(dockerBinary, "tag", "busybox", repoName)
|
|
if out, _, err := runCommandWithOutput(tagCmd); err != nil {
|
|
t.Fatalf("image tagging failed: %s, %v", out, err)
|
|
}
|
|
defer deleteImages(repoName)
|
|
|
|
pushCmd := exec.Command(dockerBinary, "push", repoName)
|
|
if out, _, err := runCommandWithOutput(pushCmd); err != nil {
|
|
t.Fatalf("pushing the image to the private registry has failed: %s, %v", out, err)
|
|
}
|
|
logDone("push - busybox to private registry")
|
|
}
|
|
|
|
// pushing an image without a prefix should throw an error
|
|
func TestPushUnprefixedRepo(t *testing.T) {
|
|
pushCmd := exec.Command(dockerBinary, "push", "busybox")
|
|
if out, _, err := runCommandWithOutput(pushCmd); err == nil {
|
|
t.Fatalf("pushing an unprefixed repo didn't result in a non-zero exit status: %s", out)
|
|
}
|
|
logDone("push - unprefixed busybox repo must not pass")
|
|
}
|
|
|
|
func TestPushUntagged(t *testing.T) {
|
|
defer setupRegistry(t)()
|
|
|
|
repoName := fmt.Sprintf("%v/dockercli/busybox", privateRegistryURL)
|
|
|
|
expected := "Repository does not exist"
|
|
pushCmd := exec.Command(dockerBinary, "push", repoName)
|
|
if out, _, err := runCommandWithOutput(pushCmd); err == nil {
|
|
t.Fatalf("pushing the image to the private registry should have failed: outuput %q", out)
|
|
} else if !strings.Contains(out, expected) {
|
|
t.Fatalf("pushing the image failed with an unexpected message: expected %q, got %q", expected, out)
|
|
}
|
|
logDone("push - untagged image")
|
|
}
|
|
|
|
func TestPushBadTag(t *testing.T) {
|
|
defer setupRegistry(t)()
|
|
|
|
repoName := fmt.Sprintf("%v/dockercli/busybox:latest", privateRegistryURL)
|
|
|
|
expected := "does not exist"
|
|
pushCmd := exec.Command(dockerBinary, "push", repoName)
|
|
if out, _, err := runCommandWithOutput(pushCmd); err == nil {
|
|
t.Fatalf("pushing the image to the private registry should have failed: outuput %q", out)
|
|
} else if !strings.Contains(out, expected) {
|
|
t.Fatalf("pushing the image failed with an unexpected message: expected %q, got %q", expected, out)
|
|
}
|
|
logDone("push - image with bad tag")
|
|
}
|
|
|
|
func TestPushMultipleTags(t *testing.T) {
|
|
defer setupRegistry(t)()
|
|
|
|
repoName := fmt.Sprintf("%v/dockercli/busybox", privateRegistryURL)
|
|
repoTag1 := fmt.Sprintf("%v/dockercli/busybox:t1", privateRegistryURL)
|
|
repoTag2 := fmt.Sprintf("%v/dockercli/busybox:t2", privateRegistryURL)
|
|
// tag the image to upload it tot he private registry
|
|
tagCmd1 := exec.Command(dockerBinary, "tag", "busybox", repoTag1)
|
|
if out, _, err := runCommandWithOutput(tagCmd1); err != nil {
|
|
t.Fatalf("image tagging failed: %s, %v", out, err)
|
|
}
|
|
defer deleteImages(repoTag1)
|
|
tagCmd2 := exec.Command(dockerBinary, "tag", "busybox", repoTag2)
|
|
if out, _, err := runCommandWithOutput(tagCmd2); err != nil {
|
|
t.Fatalf("image tagging failed: %s, %v", out, err)
|
|
}
|
|
defer deleteImages(repoTag2)
|
|
|
|
pushCmd := exec.Command(dockerBinary, "push", repoName)
|
|
if out, _, err := runCommandWithOutput(pushCmd); err != nil {
|
|
t.Fatalf("pushing the image to the private registry has failed: %s, %v", out, err)
|
|
}
|
|
logDone("push - multiple tags to private registry")
|
|
}
|
|
|
|
func TestPushInterrupt(t *testing.T) {
|
|
defer setupRegistry(t)()
|
|
|
|
repoName := fmt.Sprintf("%v/dockercli/busybox", privateRegistryURL)
|
|
// tag the image to upload it tot he private registry
|
|
tagCmd := exec.Command(dockerBinary, "tag", "busybox", repoName)
|
|
if out, _, err := runCommandWithOutput(tagCmd); err != nil {
|
|
t.Fatalf("image tagging failed: %s, %v", out, err)
|
|
}
|
|
defer deleteImages(repoName)
|
|
|
|
pushCmd := exec.Command(dockerBinary, "push", repoName)
|
|
if err := pushCmd.Start(); err != nil {
|
|
t.Fatalf("Failed to start pushing to private registry: %v", err)
|
|
}
|
|
|
|
// Interrupt push (yes, we have no idea at what point it will get killed).
|
|
time.Sleep(200 * time.Millisecond)
|
|
if err := pushCmd.Process.Kill(); err != nil {
|
|
t.Fatalf("Failed to kill push process: %v", err)
|
|
}
|
|
// Try agin
|
|
pushCmd = exec.Command(dockerBinary, "push", repoName)
|
|
if err := pushCmd.Start(); err != nil {
|
|
t.Fatalf("Failed to start pushing to private registry: %v", err)
|
|
}
|
|
|
|
logDone("push - interrupted")
|
|
}
|
|
|
|
func TestPushEmptyLayer(t *testing.T) {
|
|
defer setupRegistry(t)()
|
|
repoName := fmt.Sprintf("%v/dockercli/emptylayer", privateRegistryURL)
|
|
emptyTarball, err := ioutil.TempFile("", "empty_tarball")
|
|
if err != nil {
|
|
t.Fatalf("Unable to create test file: %v", err)
|
|
}
|
|
tw := tar.NewWriter(emptyTarball)
|
|
err = tw.Close()
|
|
if err != nil {
|
|
t.Fatalf("Error creating empty tarball: %v", err)
|
|
}
|
|
freader, err := os.Open(emptyTarball.Name())
|
|
if err != nil {
|
|
t.Fatalf("Could not open test tarball: %v", err)
|
|
}
|
|
|
|
importCmd := exec.Command(dockerBinary, "import", "-", repoName)
|
|
importCmd.Stdin = freader
|
|
out, _, err := runCommandWithOutput(importCmd)
|
|
if err != nil {
|
|
t.Errorf("import failed with errors: %v, output: %q", err, out)
|
|
}
|
|
|
|
// Now verify we can push it
|
|
pushCmd := exec.Command(dockerBinary, "push", repoName)
|
|
if out, _, err := runCommandWithOutput(pushCmd); err != nil {
|
|
t.Fatalf("pushing the image to the private registry has failed: %s, %v", out, err)
|
|
}
|
|
logDone("push - empty layer config to private registry")
|
|
}
|