mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
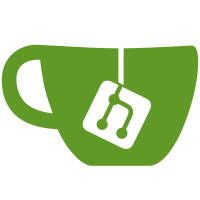
Daemon flags that can be specified multiple times use
singlar names for flags, but plural names for the configuration
file.
To make the daemon configuration know how to correlate
the flag with the corresponding configuration option,
`opt.NewNamedListOptsRef()` should be used instead of
`opt.NewListOptsRef()`.
Commit 6702ac590e
attempted
to fix the daemon not corresponding the flag with the configuration
file option, but did so by changing the name of the flag
to plural.
This patch reverts that change, and uses `opt.NewNamedListOptsRef()`
instead.
Signed-off-by: Sebastiaan van Stijn <github@gone.nl>
99 lines
6.1 KiB
Go
99 lines
6.1 KiB
Go
package main
|
|
|
|
import (
|
|
"runtime"
|
|
|
|
"github.com/docker/docker/daemon/config"
|
|
"github.com/docker/docker/opts"
|
|
"github.com/docker/docker/registry"
|
|
"github.com/spf13/pflag"
|
|
)
|
|
|
|
const (
|
|
// defaultShutdownTimeout is the default shutdown timeout for the daemon
|
|
defaultShutdownTimeout = 15
|
|
// defaultTrustKeyFile is the default filename for the trust key
|
|
defaultTrustKeyFile = "key.json"
|
|
)
|
|
|
|
// installCommonConfigFlags adds flags to the pflag.FlagSet to configure the daemon
|
|
func installCommonConfigFlags(conf *config.Config, flags *pflag.FlagSet) {
|
|
var maxConcurrentDownloads, maxConcurrentUploads int
|
|
|
|
installRegistryServiceFlags(&conf.ServiceOptions, flags)
|
|
|
|
flags.Var(opts.NewNamedListOptsRef("storage-opts", &conf.GraphOptions, nil), "storage-opt", "Storage driver options")
|
|
flags.Var(opts.NewNamedListOptsRef("authorization-plugins", &conf.AuthorizationPlugins, nil), "authorization-plugin", "Authorization plugins to load")
|
|
flags.Var(opts.NewNamedListOptsRef("exec-opts", &conf.ExecOptions, nil), "exec-opt", "Runtime execution options")
|
|
flags.StringVarP(&conf.Pidfile, "pidfile", "p", defaultPidFile, "Path to use for daemon PID file")
|
|
flags.StringVarP(&conf.Root, "graph", "g", defaultDataRoot, "Root of the Docker runtime")
|
|
flags.StringVar(&conf.ExecRoot, "exec-root", defaultExecRoot, "Root directory for execution state files")
|
|
flags.StringVar(&conf.ContainerdAddr, "containerd", "", "containerd grpc address")
|
|
|
|
// "--graph" is "soft-deprecated" in favor of "data-root". This flag was added
|
|
// before Docker 1.0, so won't be removed, only hidden, to discourage its usage.
|
|
flags.MarkHidden("graph")
|
|
|
|
flags.StringVar(&conf.Root, "data-root", defaultDataRoot, "Root directory of persistent Docker state")
|
|
|
|
flags.BoolVarP(&conf.AutoRestart, "restart", "r", true, "--restart on the daemon has been deprecated in favor of --restart policies on docker run")
|
|
flags.MarkDeprecated("restart", "Please use a restart policy on docker run")
|
|
|
|
// Windows doesn't support setting the storage driver - there is no choice as to which ones to use.
|
|
if runtime.GOOS != "windows" {
|
|
flags.StringVarP(&conf.GraphDriver, "storage-driver", "s", "", "Storage driver to use")
|
|
}
|
|
|
|
flags.IntVar(&conf.Mtu, "mtu", 0, "Set the containers network MTU")
|
|
flags.BoolVar(&conf.RawLogs, "raw-logs", false, "Full timestamps without ANSI coloring")
|
|
flags.Var(opts.NewListOptsRef(&conf.DNS, opts.ValidateIPAddress), "dns", "DNS server to use")
|
|
flags.Var(opts.NewNamedListOptsRef("dns-opts", &conf.DNSOptions, nil), "dns-opt", "DNS options to use")
|
|
flags.Var(opts.NewListOptsRef(&conf.DNSSearch, opts.ValidateDNSSearch), "dns-search", "DNS search domains to use")
|
|
flags.Var(opts.NewNamedListOptsRef("labels", &conf.Labels, opts.ValidateLabel), "label", "Set key=value labels to the daemon")
|
|
flags.StringVar(&conf.LogConfig.Type, "log-driver", "json-file", "Default driver for container logs")
|
|
flags.Var(opts.NewNamedMapOpts("log-opts", conf.LogConfig.Config, nil), "log-opt", "Default log driver options for containers")
|
|
flags.StringVar(&conf.ClusterAdvertise, "cluster-advertise", "", "Address or interface name to advertise")
|
|
flags.StringVar(&conf.ClusterStore, "cluster-store", "", "URL of the distributed storage backend")
|
|
flags.Var(opts.NewNamedMapOpts("cluster-store-opts", conf.ClusterOpts, nil), "cluster-store-opt", "Set cluster store options")
|
|
flags.StringVar(&conf.CorsHeaders, "api-cors-header", "", "Set CORS headers in the Engine API")
|
|
flags.IntVar(&maxConcurrentDownloads, "max-concurrent-downloads", config.DefaultMaxConcurrentDownloads, "Set the max concurrent downloads for each pull")
|
|
flags.IntVar(&maxConcurrentUploads, "max-concurrent-uploads", config.DefaultMaxConcurrentUploads, "Set the max concurrent uploads for each push")
|
|
flags.IntVar(&conf.ShutdownTimeout, "shutdown-timeout", defaultShutdownTimeout, "Set the default shutdown timeout")
|
|
flags.IntVar(&conf.NetworkDiagnosticPort, "network-diagnostic-port", 0, "TCP port number of the network diagnostic server")
|
|
flags.MarkHidden("network-diagnostic-port")
|
|
|
|
flags.StringVar(&conf.SwarmDefaultAdvertiseAddr, "swarm-default-advertise-addr", "", "Set default address or interface for swarm advertised address")
|
|
flags.BoolVar(&conf.Experimental, "experimental", false, "Enable experimental features")
|
|
|
|
flags.StringVar(&conf.MetricsAddress, "metrics-addr", "", "Set default address and port to serve the metrics api on")
|
|
|
|
flags.Var(opts.NewNamedListOptsRef("node-generic-resources", &conf.NodeGenericResources, opts.ValidateSingleGenericResource), "node-generic-resource", "Advertise user-defined resource")
|
|
|
|
flags.IntVar(&conf.NetworkControlPlaneMTU, "network-control-plane-mtu", config.DefaultNetworkMtu, "Network Control plane MTU")
|
|
|
|
// "--deprecated-key-path" is to allow configuration of the key used
|
|
// for the daemon ID and the deprecated image signing. It was never
|
|
// exposed as a command line option but is added here to allow
|
|
// overriding the default path in configuration.
|
|
flags.Var(opts.NewQuotedString(&conf.TrustKeyPath), "deprecated-key-path", "Path to key file for ID and image signing")
|
|
flags.MarkHidden("deprecated-key-path")
|
|
|
|
conf.MaxConcurrentDownloads = &maxConcurrentDownloads
|
|
conf.MaxConcurrentUploads = &maxConcurrentUploads
|
|
}
|
|
|
|
func installRegistryServiceFlags(options *registry.ServiceOptions, flags *pflag.FlagSet) {
|
|
ana := opts.NewNamedListOptsRef("allow-nondistributable-artifacts", &options.AllowNondistributableArtifacts, registry.ValidateIndexName)
|
|
mirrors := opts.NewNamedListOptsRef("registry-mirrors", &options.Mirrors, registry.ValidateMirror)
|
|
insecureRegistries := opts.NewNamedListOptsRef("insecure-registries", &options.InsecureRegistries, registry.ValidateIndexName)
|
|
|
|
flags.Var(ana, "allow-nondistributable-artifacts", "Allow push of nondistributable artifacts to registry")
|
|
flags.Var(mirrors, "registry-mirror", "Preferred Docker registry mirror")
|
|
flags.Var(insecureRegistries, "insecure-registry", "Enable insecure registry communication")
|
|
|
|
if runtime.GOOS != "windows" {
|
|
// TODO: Remove this flag after 3 release cycles (18.03)
|
|
flags.BoolVar(&options.V2Only, "disable-legacy-registry", true, "Disable contacting legacy registries")
|
|
flags.MarkHidden("disable-legacy-registry")
|
|
}
|
|
}
|