mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
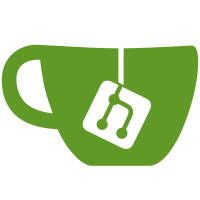
In this way, we can restore the Terminal as soon as possible once the hijacked connection end. This not only fix weird output if cli enable -D, but also remove duplicate code. Signed-off-by: Lei Jitang <leijitang@huawei.com>
93 lines
2.1 KiB
Go
93 lines
2.1 KiB
Go
package client
|
|
|
|
import (
|
|
"io"
|
|
"sync"
|
|
|
|
"golang.org/x/net/context"
|
|
|
|
"github.com/Sirupsen/logrus"
|
|
"github.com/docker/docker/pkg/stdcopy"
|
|
"github.com/docker/engine-api/types"
|
|
)
|
|
|
|
func (cli *DockerCli) holdHijackedConnection(ctx context.Context, tty bool, inputStream io.ReadCloser, outputStream, errorStream io.Writer, resp types.HijackedResponse) error {
|
|
var (
|
|
err error
|
|
restoreOnce sync.Once
|
|
)
|
|
if inputStream != nil && tty {
|
|
if err := cli.setRawTerminal(); err != nil {
|
|
return err
|
|
}
|
|
defer func() {
|
|
restoreOnce.Do(func() {
|
|
cli.restoreTerminal(inputStream)
|
|
})
|
|
}()
|
|
}
|
|
|
|
receiveStdout := make(chan error, 1)
|
|
if outputStream != nil || errorStream != nil {
|
|
go func() {
|
|
// When TTY is ON, use regular copy
|
|
if tty && outputStream != nil {
|
|
_, err = io.Copy(outputStream, resp.Reader)
|
|
// we should restore the terminal as soon as possible once connection end
|
|
// so any following print messages will be in normal type.
|
|
if inputStream != nil {
|
|
restoreOnce.Do(func() {
|
|
cli.restoreTerminal(inputStream)
|
|
})
|
|
}
|
|
} else {
|
|
_, err = stdcopy.StdCopy(outputStream, errorStream, resp.Reader)
|
|
}
|
|
|
|
logrus.Debugf("[hijack] End of stdout")
|
|
receiveStdout <- err
|
|
}()
|
|
}
|
|
|
|
stdinDone := make(chan struct{})
|
|
go func() {
|
|
if inputStream != nil {
|
|
io.Copy(resp.Conn, inputStream)
|
|
// we should restore the terminal as soon as possible once connection end
|
|
// so any following print messages will be in normal type.
|
|
if tty {
|
|
restoreOnce.Do(func() {
|
|
cli.restoreTerminal(inputStream)
|
|
})
|
|
}
|
|
logrus.Debugf("[hijack] End of stdin")
|
|
}
|
|
|
|
if err := resp.CloseWrite(); err != nil {
|
|
logrus.Debugf("Couldn't send EOF: %s", err)
|
|
}
|
|
close(stdinDone)
|
|
}()
|
|
|
|
select {
|
|
case err := <-receiveStdout:
|
|
if err != nil {
|
|
logrus.Debugf("Error receiveStdout: %s", err)
|
|
return err
|
|
}
|
|
case <-stdinDone:
|
|
if outputStream != nil || errorStream != nil {
|
|
select {
|
|
case err := <-receiveStdout:
|
|
if err != nil {
|
|
logrus.Debugf("Error receiveStdout: %s", err)
|
|
return err
|
|
}
|
|
case <-ctx.Done():
|
|
}
|
|
}
|
|
case <-ctx.Done():
|
|
}
|
|
|
|
return nil
|
|
}
|