mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
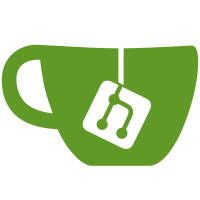
The configuration format for docker runtime is based on daemon flags and hence adjusting the libnetwork configuration to accomodate it by moving the TOML based configuration to the dnet tool. Also changed the controller configuration via options Signed-off-by: Madhu Venugopal <madhu@docker.com>
51 lines
1.4 KiB
Go
51 lines
1.4 KiB
Go
package main
|
|
|
|
import (
|
|
"fmt"
|
|
"os"
|
|
|
|
flag "github.com/docker/docker/pkg/mflag"
|
|
)
|
|
|
|
type command struct {
|
|
name string
|
|
description string
|
|
}
|
|
|
|
type byName []command
|
|
|
|
var (
|
|
flDaemon = flag.Bool([]string{"d", "-daemon"}, false, "Enable daemon mode")
|
|
flHost = flag.String([]string{"H", "-host"}, "", "Daemon socket to connect to")
|
|
flLogLevel = flag.String([]string{"l", "-log-level"}, "info", "Set the logging level")
|
|
flDebug = flag.Bool([]string{"D", "-debug"}, false, "Enable debug mode")
|
|
flCfgFile = flag.String([]string{"c", "-cfg-file"}, "/etc/default/libnetwork.toml", "Configuration file")
|
|
flHelp = flag.Bool([]string{"h", "-help"}, false, "Print usage")
|
|
|
|
dnetCommands = []command{
|
|
{"network", "Network management commands"},
|
|
{"service", "Service management commands"},
|
|
}
|
|
)
|
|
|
|
func init() {
|
|
flag.Usage = func() {
|
|
fmt.Fprint(os.Stdout, "Usage: dnet [OPTIONS] COMMAND [arg...]\n\nA self-sufficient runtime for container networking.\n\nOptions:\n")
|
|
|
|
flag.CommandLine.SetOutput(os.Stdout)
|
|
flag.PrintDefaults()
|
|
|
|
help := "\nCommands:\n"
|
|
|
|
for _, cmd := range dnetCommands {
|
|
help += fmt.Sprintf(" %-10.10s%s\n", cmd.name, cmd.description)
|
|
}
|
|
|
|
help += "\nRun 'dnet COMMAND --help' for more information on a command."
|
|
fmt.Fprintf(os.Stdout, "%s\n", help)
|
|
}
|
|
}
|
|
|
|
func printUsage() {
|
|
fmt.Println("Usage: dnet <OPTIONS> COMMAND [arg...]")
|
|
}
|