mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
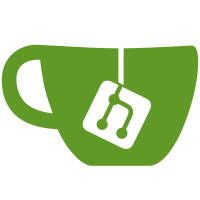
github.com/gotestyourself/gotestyourself moved to gotest.tools with version 2.0.0. Moving to that one, bumping it to v2.1.0. Signed-off-by: Vincent Demeester <vincent@sbr.pm>
32 lines
768 B
Go
32 lines
768 B
Go
package icmd
|
|
|
|
import (
|
|
"os/exec"
|
|
"syscall"
|
|
|
|
"github.com/pkg/errors"
|
|
)
|
|
|
|
// getExitCode returns the ExitStatus of a process from the error returned by
|
|
// exec.Run(). If the exit status could not be parsed an error is returned.
|
|
func getExitCode(err error) (int, error) {
|
|
if exiterr, ok := err.(*exec.ExitError); ok {
|
|
if procExit, ok := exiterr.Sys().(syscall.WaitStatus); ok {
|
|
return procExit.ExitStatus(), nil
|
|
}
|
|
}
|
|
return 0, errors.Wrap(err, "failed to get exit code")
|
|
}
|
|
|
|
func processExitCode(err error) (exitCode int) {
|
|
if err == nil {
|
|
return 0
|
|
}
|
|
exitCode, exiterr := getExitCode(err)
|
|
if exiterr != nil {
|
|
// TODO: Fix this so we check the error's text.
|
|
// we've failed to retrieve exit code, so we set it to 127
|
|
return 127
|
|
}
|
|
return exitCode
|
|
}
|