mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
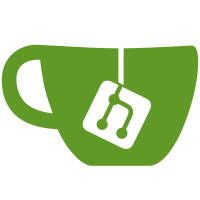
All other endpoints handle this in the API; given that the JSON format for filters is part of the API, it makes sense to handle it there, and not have that concept leak into further down the code. Signed-off-by: Sebastiaan van Stijn <github@gone.nl>
41 lines
1.8 KiB
Go
41 lines
1.8 KiB
Go
package image // import "github.com/docker/docker/api/server/router/image"
|
|
|
|
import (
|
|
"context"
|
|
"io"
|
|
|
|
"github.com/docker/docker/api/types"
|
|
"github.com/docker/docker/api/types/filters"
|
|
"github.com/docker/docker/api/types/image"
|
|
"github.com/docker/docker/api/types/registry"
|
|
specs "github.com/opencontainers/image-spec/specs-go/v1"
|
|
)
|
|
|
|
// Backend is all the methods that need to be implemented
|
|
// to provide image specific functionality.
|
|
type Backend interface {
|
|
imageBackend
|
|
importExportBackend
|
|
registryBackend
|
|
}
|
|
|
|
type imageBackend interface {
|
|
ImageDelete(imageRef string, force, prune bool) ([]types.ImageDeleteResponseItem, error)
|
|
ImageHistory(imageName string) ([]*image.HistoryResponseItem, error)
|
|
Images(ctx context.Context, opts types.ImageListOptions) ([]*types.ImageSummary, error)
|
|
LookupImage(name string) (*types.ImageInspect, error)
|
|
TagImage(imageName, repository, tag string) (string, error)
|
|
ImagesPrune(ctx context.Context, pruneFilters filters.Args) (*types.ImagesPruneReport, error)
|
|
}
|
|
|
|
type importExportBackend interface {
|
|
LoadImage(inTar io.ReadCloser, outStream io.Writer, quiet bool) error
|
|
ImportImage(src string, repository string, platform *specs.Platform, tag string, msg string, inConfig io.ReadCloser, outStream io.Writer, changes []string) error
|
|
ExportImage(names []string, outStream io.Writer) error
|
|
}
|
|
|
|
type registryBackend interface {
|
|
PullImage(ctx context.Context, image, tag string, platform *specs.Platform, metaHeaders map[string][]string, authConfig *types.AuthConfig, outStream io.Writer) error
|
|
PushImage(ctx context.Context, image, tag string, metaHeaders map[string][]string, authConfig *types.AuthConfig, outStream io.Writer) error
|
|
SearchRegistryForImages(ctx context.Context, searchFilters filters.Args, term string, limit int, authConfig *types.AuthConfig, metaHeaders map[string][]string) (*registry.SearchResults, error)
|
|
}
|