mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
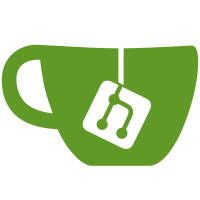
This changes the default ipc mode of daemon/engine to be private, meaning the containers will not have their /dev/shm bind-mounted from the host by default. The benefits of doing this are: 1. No leaked mounts. Eliminate a possibility to leak mounts into other namespaces (and therefore unfortunate errors like "Unable to remove filesystem for <ID>: remove /var/lib/docker/containers/<ID>/shm: device or resource busy"). 2. Working checkpoint/restore. Make `docker checkpoint` not lose the contents of `/dev/shm`, but save it to the dump, and be restored back upon `docker start --checkpoint` (currently it is lost -- while CRIU handles tmpfs mounts, the "shareable" mount is seen as external to container, and thus rightfully ignored). 3. Better security. Currently any container is opened to share its /dev/shm with any other container. Obviously, this change will break the following usage scenario: $ docker run -d --name donor busybox top $ docker run --rm -it --ipc container:donor busybox sh Error response from daemon: linux spec namespaces: can't join IPC of container <ID>: non-shareable IPC (hint: use IpcMode:shareable for the donor container) The soution, as hinted by the (amended) error message, is to explicitly enable donor sharing by using --ipc shareable: $ docker run -d --name donor --ipc shareable busybox top Compatibility notes: 1. This only applies to containers created _after_ this change. Existing containers are not affected and will work fine as their ipc mode is stored in HostConfig. 2. Old backward compatible behavior ("shareable" containers by default) can be enabled by either using `--default-ipc-mode shareable` daemon command line option, or by adding a `"default-ipc-mode": "shareable"` line in `/etc/docker/daemon.json` configuration file. 3. If an older client (API < 1.40) is used, a "shareable" container is created. A test to check that is added. Signed-off-by: Kir Kolyshkin <kolyshkin@gmail.com>
95 lines
3.6 KiB
Go
95 lines
3.6 KiB
Go
// +build linux freebsd
|
|
|
|
package config // import "github.com/docker/docker/daemon/config"
|
|
|
|
import (
|
|
"fmt"
|
|
|
|
containertypes "github.com/docker/docker/api/types/container"
|
|
"github.com/docker/docker/opts"
|
|
"github.com/docker/go-units"
|
|
)
|
|
|
|
const (
|
|
// DefaultIpcMode is default for container's IpcMode, if not set otherwise
|
|
DefaultIpcMode = "private"
|
|
)
|
|
|
|
// Config defines the configuration of a docker daemon.
|
|
// It includes json tags to deserialize configuration from a file
|
|
// using the same names that the flags in the command line uses.
|
|
type Config struct {
|
|
CommonConfig
|
|
|
|
// These fields are common to all unix platforms.
|
|
CommonUnixConfig
|
|
// Fields below here are platform specific.
|
|
CgroupParent string `json:"cgroup-parent,omitempty"`
|
|
EnableSelinuxSupport bool `json:"selinux-enabled,omitempty"`
|
|
RemappedRoot string `json:"userns-remap,omitempty"`
|
|
Ulimits map[string]*units.Ulimit `json:"default-ulimits,omitempty"`
|
|
CPURealtimePeriod int64 `json:"cpu-rt-period,omitempty"`
|
|
CPURealtimeRuntime int64 `json:"cpu-rt-runtime,omitempty"`
|
|
OOMScoreAdjust int `json:"oom-score-adjust,omitempty"`
|
|
Init bool `json:"init,omitempty"`
|
|
InitPath string `json:"init-path,omitempty"`
|
|
SeccompProfile string `json:"seccomp-profile,omitempty"`
|
|
ShmSize opts.MemBytes `json:"default-shm-size,omitempty"`
|
|
NoNewPrivileges bool `json:"no-new-privileges,omitempty"`
|
|
IpcMode string `json:"default-ipc-mode,omitempty"`
|
|
// ResolvConf is the path to the configuration of the host resolver
|
|
ResolvConf string `json:"resolv-conf,omitempty"`
|
|
Rootless bool `json:"rootless,omitempty"`
|
|
}
|
|
|
|
// BridgeConfig stores all the bridge driver specific
|
|
// configuration.
|
|
type BridgeConfig struct {
|
|
commonBridgeConfig
|
|
|
|
// These fields are common to all unix platforms.
|
|
commonUnixBridgeConfig
|
|
|
|
// Fields below here are platform specific.
|
|
EnableIPv6 bool `json:"ipv6,omitempty"`
|
|
EnableIPTables bool `json:"iptables,omitempty"`
|
|
EnableIPForward bool `json:"ip-forward,omitempty"`
|
|
EnableIPMasq bool `json:"ip-masq,omitempty"`
|
|
EnableUserlandProxy bool `json:"userland-proxy,omitempty"`
|
|
UserlandProxyPath string `json:"userland-proxy-path,omitempty"`
|
|
FixedCIDRv6 string `json:"fixed-cidr-v6,omitempty"`
|
|
}
|
|
|
|
// IsSwarmCompatible defines if swarm mode can be enabled in this config
|
|
func (conf *Config) IsSwarmCompatible() error {
|
|
if conf.ClusterStore != "" || conf.ClusterAdvertise != "" {
|
|
return fmt.Errorf("--cluster-store and --cluster-advertise daemon configurations are incompatible with swarm mode")
|
|
}
|
|
if conf.LiveRestoreEnabled {
|
|
return fmt.Errorf("--live-restore daemon configuration is incompatible with swarm mode")
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func verifyDefaultIpcMode(mode string) error {
|
|
const hint = "Use \"shareable\" or \"private\"."
|
|
|
|
dm := containertypes.IpcMode(mode)
|
|
if !dm.Valid() {
|
|
return fmt.Errorf("Default IPC mode setting (%v) is invalid. "+hint, dm)
|
|
}
|
|
if dm != "" && !dm.IsPrivate() && !dm.IsShareable() {
|
|
return fmt.Errorf("IPC mode \"%v\" is not supported as default value. "+hint, dm)
|
|
}
|
|
return nil
|
|
}
|
|
|
|
// ValidatePlatformConfig checks if any platform-specific configuration settings are invalid.
|
|
func (conf *Config) ValidatePlatformConfig() error {
|
|
return verifyDefaultIpcMode(conf.IpcMode)
|
|
}
|
|
|
|
// IsRootless returns conf.Rootless
|
|
func (conf *Config) IsRootless() bool {
|
|
return conf.Rootless
|
|
}
|