mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
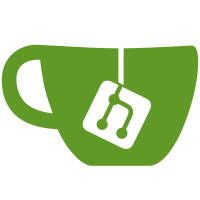
`docker rename foo ''` would result in: ``` usage: docker rename OLD_NAME NEW_NAME ``` which is the old engine's way of return errors - yes that's in the daemon code. So I fixed that error msg to just be normal. While doing that I noticed that using an empty string for the source container name failed but didn't print any error message at all. This is because we would generate a URL like: ../containers//rename/.. which would cause a 301 redirect to ../containers/rename/.. however the CLI code doesn't actually deal with 301's - it just ignores them and returns back to the CLI code/caller. Rather than changing the CLI to deal with 3xx error codes, which would probably be a good thing to do in a follow-on PR, for this immediate issue I just added a cli-side check for empty strings for both old and new names. This way we catch it even before we hit the daemon. API callers will get a 404, assuming they follow the 301, for the case of the src being empty, and the new error msg when the destination is empty - so we should be good now. Add tests for both cases too. Signed-off-by: Doug Davis <dug@us.ibm.com>
32 lines
862 B
Go
32 lines
862 B
Go
package client
|
|
|
|
import (
|
|
"fmt"
|
|
"strings"
|
|
|
|
Cli "github.com/docker/docker/cli"
|
|
flag "github.com/docker/docker/pkg/mflag"
|
|
)
|
|
|
|
// CmdRename renames a container.
|
|
//
|
|
// Usage: docker rename OLD_NAME NEW_NAME
|
|
func (cli *DockerCli) CmdRename(args ...string) error {
|
|
cmd := Cli.Subcmd("rename", []string{"OLD_NAME NEW_NAME"}, "Rename a container", true)
|
|
cmd.Require(flag.Exact, 2)
|
|
|
|
cmd.ParseFlags(args, true)
|
|
|
|
oldName := strings.TrimSpace(cmd.Arg(0))
|
|
newName := strings.TrimSpace(cmd.Arg(1))
|
|
|
|
if oldName == "" || newName == "" {
|
|
return fmt.Errorf("Error: Neither old nor new names may be empty")
|
|
}
|
|
|
|
if _, _, err := readBody(cli.call("POST", fmt.Sprintf("/containers/%s/rename?name=%s", oldName, newName), nil, nil)); err != nil {
|
|
fmt.Fprintf(cli.err, "%s\n", err)
|
|
return fmt.Errorf("Error: failed to rename container named %s", oldName)
|
|
}
|
|
return nil
|
|
}
|