mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
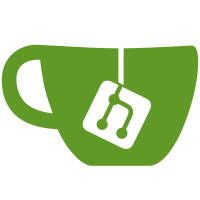
When plugins have a positive refcount, they were not allowed to be removed. However, plugins could still be disabled when volumes referenced it and containers using them were running. This change fixes that by enforcing plugin refcount during disable. A "force" disable option is also added to ignore reference refcounting. Signed-off-by: Anusha Ragunathan <anusha@docker.com>
48 lines
1.2 KiB
Go
48 lines
1.2 KiB
Go
package client
|
|
|
|
import (
|
|
"bytes"
|
|
"fmt"
|
|
"io/ioutil"
|
|
"net/http"
|
|
"strings"
|
|
"testing"
|
|
|
|
"github.com/docker/docker/api/types"
|
|
"golang.org/x/net/context"
|
|
)
|
|
|
|
func TestPluginDisableError(t *testing.T) {
|
|
client := &Client{
|
|
client: newMockClient(errorMock(http.StatusInternalServerError, "Server error")),
|
|
}
|
|
|
|
err := client.PluginDisable(context.Background(), "plugin_name", types.PluginDisableOptions{})
|
|
if err == nil || err.Error() != "Error response from daemon: Server error" {
|
|
t.Fatalf("expected a Server Error, got %v", err)
|
|
}
|
|
}
|
|
|
|
func TestPluginDisable(t *testing.T) {
|
|
expectedURL := "/plugins/plugin_name/disable"
|
|
|
|
client := &Client{
|
|
client: newMockClient(func(req *http.Request) (*http.Response, error) {
|
|
if !strings.HasPrefix(req.URL.Path, expectedURL) {
|
|
return nil, fmt.Errorf("Expected URL '%s', got '%s'", expectedURL, req.URL)
|
|
}
|
|
if req.Method != "POST" {
|
|
return nil, fmt.Errorf("expected POST method, got %s", req.Method)
|
|
}
|
|
return &http.Response{
|
|
StatusCode: http.StatusOK,
|
|
Body: ioutil.NopCloser(bytes.NewReader([]byte(""))),
|
|
}, nil
|
|
}),
|
|
}
|
|
|
|
err := client.PluginDisable(context.Background(), "plugin_name", types.PluginDisableOptions{})
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
}
|