mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
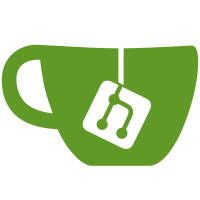
The image store abstracts image handling. It keeps track of the available images, and makes it possible to delete existing images or register new ones. The image store holds references to the underlying layers for each image. The image/v1 package provides compatibility functions for interoperating with older (non-content-addressable) image structures. Signed-off-by: Tonis Tiigi <tonistiigi@gmail.com>
59 lines
1.1 KiB
Go
59 lines
1.1 KiB
Go
package image
|
|
|
|
import (
|
|
"encoding/json"
|
|
"sort"
|
|
"strings"
|
|
"testing"
|
|
)
|
|
|
|
const sampleImageJSON = `{
|
|
"architecture": "amd64",
|
|
"os": "linux",
|
|
"config": {},
|
|
"rootfs": {
|
|
"type": "layers",
|
|
"diff_ids": []
|
|
}
|
|
}`
|
|
|
|
func TestJSON(t *testing.T) {
|
|
img, err := NewFromJSON([]byte(sampleImageJSON))
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
rawJSON := img.RawJSON()
|
|
if string(rawJSON) != sampleImageJSON {
|
|
t.Fatalf("Raw JSON of config didn't match: expected %+v, got %v", sampleImageJSON, rawJSON)
|
|
}
|
|
}
|
|
|
|
func TestInvalidJSON(t *testing.T) {
|
|
_, err := NewFromJSON([]byte("{}"))
|
|
if err == nil {
|
|
t.Fatal("Expected JSON parse error")
|
|
}
|
|
}
|
|
|
|
func TestMarshalKeyOrder(t *testing.T) {
|
|
b, err := json.Marshal(&Image{
|
|
V1Image: V1Image{
|
|
Comment: "a",
|
|
Author: "b",
|
|
Architecture: "c",
|
|
},
|
|
})
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
|
|
expectedOrder := []string{"architecture", "author", "comment"}
|
|
var indexes []int
|
|
for _, k := range expectedOrder {
|
|
indexes = append(indexes, strings.Index(string(b), k))
|
|
}
|
|
|
|
if !sort.IntsAreSorted(indexes) {
|
|
t.Fatal("invalid key order in JSON: ", string(b))
|
|
}
|
|
}
|