mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
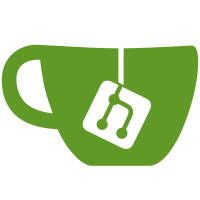
Currently, the resources associated with the io.Reader returned by TarStream are only freed when it is read until EOF. This means that partial uploads or exports (for example, in the case of a full disk or severed connection) can leak a goroutine and open file. This commit changes TarStream to return an io.ReadCloser. Resources are freed when Close is called. Signed-off-by: Aaron Lehmann <aaron.lehmann@docker.com>
48 lines
1 KiB
Go
48 lines
1 KiB
Go
package layer
|
|
|
|
import (
|
|
"archive/tar"
|
|
"bytes"
|
|
"io"
|
|
"io/ioutil"
|
|
)
|
|
|
|
// DigestSHA256EmptyTar is the canonical sha256 digest of empty tar file -
|
|
// (1024 NULL bytes)
|
|
const DigestSHA256EmptyTar = DiffID("sha256:5f70bf18a086007016e948b04aed3b82103a36bea41755b6cddfaf10ace3c6ef")
|
|
|
|
type emptyLayer struct{}
|
|
|
|
// EmptyLayer is a layer that corresponds to empty tar.
|
|
var EmptyLayer = &emptyLayer{}
|
|
|
|
func (el *emptyLayer) TarStream() (io.ReadCloser, error) {
|
|
buf := new(bytes.Buffer)
|
|
tarWriter := tar.NewWriter(buf)
|
|
tarWriter.Close()
|
|
return ioutil.NopCloser(buf), nil
|
|
}
|
|
|
|
func (el *emptyLayer) ChainID() ChainID {
|
|
return ChainID(DigestSHA256EmptyTar)
|
|
}
|
|
|
|
func (el *emptyLayer) DiffID() DiffID {
|
|
return DigestSHA256EmptyTar
|
|
}
|
|
|
|
func (el *emptyLayer) Parent() Layer {
|
|
return nil
|
|
}
|
|
|
|
func (el *emptyLayer) Size() (size int64, err error) {
|
|
return 0, nil
|
|
}
|
|
|
|
func (el *emptyLayer) DiffSize() (size int64, err error) {
|
|
return 0, nil
|
|
}
|
|
|
|
func (el *emptyLayer) Metadata() (map[string]string, error) {
|
|
return make(map[string]string), nil
|
|
}
|