mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
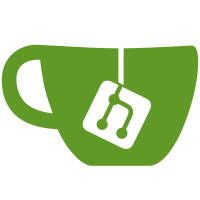
Adds functionality to parse and return network attachment spec information. Network attachment tasks are phony tasks created in swarmkit to deal with unmanaged containers attached to swarmkit. Before this change, attempting `docker inspect` on the task id of a network attachment task would result in an empty task object. After this change, a full task object is returned Fixes #26548 the correct way. Signed-off-by: Drew Erny <drew.erny@docker.com> Signed-off-by: Sebastiaan van Stijn <github@gone.nl>
69 lines
2.1 KiB
Go
69 lines
2.1 KiB
Go
package convert // import "github.com/docker/docker/daemon/cluster/convert"
|
|
|
|
import (
|
|
"strings"
|
|
|
|
types "github.com/docker/docker/api/types/swarm"
|
|
swarmapi "github.com/docker/swarmkit/api"
|
|
gogotypes "github.com/gogo/protobuf/types"
|
|
)
|
|
|
|
// TaskFromGRPC converts a grpc Task to a Task.
|
|
func TaskFromGRPC(t swarmapi.Task) (types.Task, error) {
|
|
containerStatus := t.Status.GetContainer()
|
|
taskSpec, err := taskSpecFromGRPC(t.Spec)
|
|
if err != nil {
|
|
return types.Task{}, err
|
|
}
|
|
task := types.Task{
|
|
ID: t.ID,
|
|
Annotations: annotationsFromGRPC(t.Annotations),
|
|
ServiceID: t.ServiceID,
|
|
Slot: int(t.Slot),
|
|
NodeID: t.NodeID,
|
|
Spec: taskSpec,
|
|
Status: types.TaskStatus{
|
|
State: types.TaskState(strings.ToLower(t.Status.State.String())),
|
|
Message: t.Status.Message,
|
|
Err: t.Status.Err,
|
|
},
|
|
DesiredState: types.TaskState(strings.ToLower(t.DesiredState.String())),
|
|
GenericResources: GenericResourcesFromGRPC(t.AssignedGenericResources),
|
|
}
|
|
|
|
// Meta
|
|
task.Version.Index = t.Meta.Version.Index
|
|
task.CreatedAt, _ = gogotypes.TimestampFromProto(t.Meta.CreatedAt)
|
|
task.UpdatedAt, _ = gogotypes.TimestampFromProto(t.Meta.UpdatedAt)
|
|
|
|
task.Status.Timestamp, _ = gogotypes.TimestampFromProto(t.Status.Timestamp)
|
|
|
|
if containerStatus != nil {
|
|
task.Status.ContainerStatus = &types.ContainerStatus{
|
|
ContainerID: containerStatus.ContainerID,
|
|
PID: int(containerStatus.PID),
|
|
ExitCode: int(containerStatus.ExitCode),
|
|
}
|
|
}
|
|
|
|
// NetworksAttachments
|
|
for _, na := range t.Networks {
|
|
task.NetworksAttachments = append(task.NetworksAttachments, networkAttachmentFromGRPC(na))
|
|
}
|
|
|
|
if t.Status.PortStatus == nil {
|
|
return task, nil
|
|
}
|
|
|
|
for _, p := range t.Status.PortStatus.Ports {
|
|
task.Status.PortStatus.Ports = append(task.Status.PortStatus.Ports, types.PortConfig{
|
|
Name: p.Name,
|
|
Protocol: types.PortConfigProtocol(strings.ToLower(swarmapi.PortConfig_Protocol_name[int32(p.Protocol)])),
|
|
PublishMode: types.PortConfigPublishMode(strings.ToLower(swarmapi.PortConfig_PublishMode_name[int32(p.PublishMode)])),
|
|
TargetPort: p.TargetPort,
|
|
PublishedPort: p.PublishedPort,
|
|
})
|
|
}
|
|
|
|
return task, nil
|
|
}
|