mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
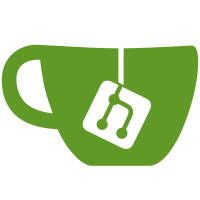
Use a tagged release of Cobra. All relevant PR's were merged, so the fork is no longer needed. Relevant changes: - spf13/cobra#552 Add a field to disable [flags] in UseLine() - spf13/cobra#567 Add `CalledAs` method to cobra.Command - spf13/cobra#580 Update error message for missing required flags - spf13/cobra#584 Add support for --version flag - spf13/cobra#614 If user has a project in symlink, just use its destination folder and work there - spf13/cobra#649 terminates the flags when -- is found in commandline - spf13/cobra#662 Add support for ignoring parse errors - spf13/cobra#686 doc: hide hidden parent flags Also various improvements were added for generating Bash completion scripts (currently not used by us) Fixes usage output for dockerd; Before this update: dockerd --help Usage: dockerd COMMAND A self-sufficient runtime for containers. After this update: dockerd --help Usage: dockerd [OPTIONS] [flags] A self-sufficient runtime for containers. Bump spf13/pflag to v1.0.1 Relevant changes: - spf13/pflag#106 allow lookup by shorthand - spf13/pflag#113 Add SortFlags option - spf13/pflag#138 Generate flag error output for errors returned from the parseFunc - spf13/pflag#141 Fixing Count flag usage string - spf13/pflag#143 add int16 flag - spf13/pflag#122 DurationSlice: implementation and tests - spf13/pflag#115 Implement BytesHex type of argument - spf13/pflag#150 Add uintSlice and boolSlice to name prettifier - spf13/pflag#155 Add multiline wrapping support - spf13/pflag#158 doc: clarify difference between string slice vs. array - spf13/pflag#160 add ability to ignore unknown flags - spf13/pflag#163 Allow Users To Show Deprecated Flags Hide [flags] in usage output Hides the [flags] in the usage output of commands (present in newer versions of Cobra), using the `.DisableFlagsInUseLine` option. Before this change: dockerd --help Usage: dockerd [OPTIONS] [flags] A self-sufficient runtime for containers. After this change: dockerd --help Usage: dockerd [OPTIONS] A self-sufficient runtime for containers. Signed-off-by: Sebastiaan van Stijn <github@gone.nl> Â# modified: vendor/github.com/spf13/pflag/string_array.go § Signed-off-by: Sebastiaan van Stijn <github@gone.nl>
88 lines
2.9 KiB
Go
88 lines
2.9 KiB
Go
package pflag
|
|
|
|
import "strconv"
|
|
|
|
// -- int16 Value
|
|
type int16Value int16
|
|
|
|
func newInt16Value(val int16, p *int16) *int16Value {
|
|
*p = val
|
|
return (*int16Value)(p)
|
|
}
|
|
|
|
func (i *int16Value) Set(s string) error {
|
|
v, err := strconv.ParseInt(s, 0, 16)
|
|
*i = int16Value(v)
|
|
return err
|
|
}
|
|
|
|
func (i *int16Value) Type() string {
|
|
return "int16"
|
|
}
|
|
|
|
func (i *int16Value) String() string { return strconv.FormatInt(int64(*i), 10) }
|
|
|
|
func int16Conv(sval string) (interface{}, error) {
|
|
v, err := strconv.ParseInt(sval, 0, 16)
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
return int16(v), nil
|
|
}
|
|
|
|
// GetInt16 returns the int16 value of a flag with the given name
|
|
func (f *FlagSet) GetInt16(name string) (int16, error) {
|
|
val, err := f.getFlagType(name, "int16", int16Conv)
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
return val.(int16), nil
|
|
}
|
|
|
|
// Int16Var defines an int16 flag with specified name, default value, and usage string.
|
|
// The argument p points to an int16 variable in which to store the value of the flag.
|
|
func (f *FlagSet) Int16Var(p *int16, name string, value int16, usage string) {
|
|
f.VarP(newInt16Value(value, p), name, "", usage)
|
|
}
|
|
|
|
// Int16VarP is like Int16Var, but accepts a shorthand letter that can be used after a single dash.
|
|
func (f *FlagSet) Int16VarP(p *int16, name, shorthand string, value int16, usage string) {
|
|
f.VarP(newInt16Value(value, p), name, shorthand, usage)
|
|
}
|
|
|
|
// Int16Var defines an int16 flag with specified name, default value, and usage string.
|
|
// The argument p points to an int16 variable in which to store the value of the flag.
|
|
func Int16Var(p *int16, name string, value int16, usage string) {
|
|
CommandLine.VarP(newInt16Value(value, p), name, "", usage)
|
|
}
|
|
|
|
// Int16VarP is like Int16Var, but accepts a shorthand letter that can be used after a single dash.
|
|
func Int16VarP(p *int16, name, shorthand string, value int16, usage string) {
|
|
CommandLine.VarP(newInt16Value(value, p), name, shorthand, usage)
|
|
}
|
|
|
|
// Int16 defines an int16 flag with specified name, default value, and usage string.
|
|
// The return value is the address of an int16 variable that stores the value of the flag.
|
|
func (f *FlagSet) Int16(name string, value int16, usage string) *int16 {
|
|
p := new(int16)
|
|
f.Int16VarP(p, name, "", value, usage)
|
|
return p
|
|
}
|
|
|
|
// Int16P is like Int16, but accepts a shorthand letter that can be used after a single dash.
|
|
func (f *FlagSet) Int16P(name, shorthand string, value int16, usage string) *int16 {
|
|
p := new(int16)
|
|
f.Int16VarP(p, name, shorthand, value, usage)
|
|
return p
|
|
}
|
|
|
|
// Int16 defines an int16 flag with specified name, default value, and usage string.
|
|
// The return value is the address of an int16 variable that stores the value of the flag.
|
|
func Int16(name string, value int16, usage string) *int16 {
|
|
return CommandLine.Int16P(name, "", value, usage)
|
|
}
|
|
|
|
// Int16P is like Int16, but accepts a shorthand letter that can be used after a single dash.
|
|
func Int16P(name, shorthand string, value int16, usage string) *int16 {
|
|
return CommandLine.Int16P(name, shorthand, value, usage)
|
|
}
|