mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
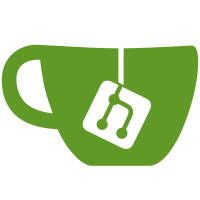
Since Go 1.7, context is a standard package. Since Go 1.9, everything that is provided by "x/net/context" is a couple of type aliases to types in "context". Many vendored packages still use x/net/context, so vendor entry remains for now. Signed-off-by: Kir Kolyshkin <kolyshkin@gmail.com>
66 lines
2.2 KiB
Go
66 lines
2.2 KiB
Go
package swarm // import "github.com/docker/docker/api/server/router/swarm"
|
|
|
|
import (
|
|
"context"
|
|
"fmt"
|
|
"io"
|
|
"net/http"
|
|
|
|
"github.com/docker/docker/api/server/httputils"
|
|
basictypes "github.com/docker/docker/api/types"
|
|
"github.com/docker/docker/api/types/backend"
|
|
)
|
|
|
|
// swarmLogs takes an http response, request, and selector, and writes the logs
|
|
// specified by the selector to the response
|
|
func (sr *swarmRouter) swarmLogs(ctx context.Context, w io.Writer, r *http.Request, selector *backend.LogSelector) error {
|
|
// Args are validated before the stream starts because when it starts we're
|
|
// sending HTTP 200 by writing an empty chunk of data to tell the client that
|
|
// daemon is going to stream. By sending this initial HTTP 200 we can't report
|
|
// any error after the stream starts (i.e. container not found, wrong parameters)
|
|
// with the appropriate status code.
|
|
stdout, stderr := httputils.BoolValue(r, "stdout"), httputils.BoolValue(r, "stderr")
|
|
if !(stdout || stderr) {
|
|
return fmt.Errorf("Bad parameters: you must choose at least one stream")
|
|
}
|
|
|
|
// there is probably a neater way to manufacture the ContainerLogsOptions
|
|
// struct, probably in the caller, to eliminate the dependency on net/http
|
|
logsConfig := &basictypes.ContainerLogsOptions{
|
|
Follow: httputils.BoolValue(r, "follow"),
|
|
Timestamps: httputils.BoolValue(r, "timestamps"),
|
|
Since: r.Form.Get("since"),
|
|
Tail: r.Form.Get("tail"),
|
|
ShowStdout: stdout,
|
|
ShowStderr: stderr,
|
|
Details: httputils.BoolValue(r, "details"),
|
|
}
|
|
|
|
tty := false
|
|
// checking for whether logs are TTY involves iterating over every service
|
|
// and task. idk if there is a better way
|
|
for _, service := range selector.Services {
|
|
s, err := sr.backend.GetService(service, false)
|
|
if err != nil {
|
|
// maybe should return some context with this error?
|
|
return err
|
|
}
|
|
tty = (s.Spec.TaskTemplate.ContainerSpec != nil && s.Spec.TaskTemplate.ContainerSpec.TTY) || tty
|
|
}
|
|
for _, task := range selector.Tasks {
|
|
t, err := sr.backend.GetTask(task)
|
|
if err != nil {
|
|
// as above
|
|
return err
|
|
}
|
|
tty = t.Spec.ContainerSpec.TTY || tty
|
|
}
|
|
|
|
msgs, err := sr.backend.ServiceLogs(ctx, selector, logsConfig)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
|
|
httputils.WriteLogStream(ctx, w, msgs, logsConfig, !tty)
|
|
return nil
|
|
}
|