mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
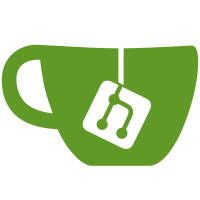
Added --selinux-enable switch to daemon to enable SELinux labeling. The daemon will now generate a new unique random SELinux label when a container starts, and remove it when the container is removed. The MCS labels will be stored in the daemon memory. The labels of containers will be stored in the container.json file. When the daemon restarts on boot or if done by an admin, it will read all containers json files and reserve the MCS labels. A potential problem would be conflicts if you setup thousands of containers, current scheme would handle ~500,000 containers. Docker-DCO-1.1-Signed-off-by: Dan Walsh <dwalsh@redhat.com> (github: rhatdan) Docker-DCO-1.1-Signed-off-by: Dan Walsh <dwalsh@redhat.com> (github: crosbymichael)
73 lines
2.3 KiB
Go
73 lines
2.3 KiB
Go
package daemonconfig
|
|
|
|
import (
|
|
"github.com/dotcloud/docker/daemon/networkdriver"
|
|
"github.com/dotcloud/docker/engine"
|
|
"net"
|
|
)
|
|
|
|
const (
|
|
defaultNetworkMtu = 1500
|
|
DisableNetworkBridge = "none"
|
|
)
|
|
|
|
// FIXME: separate runtime configuration from http api configuration
|
|
type Config struct {
|
|
Pidfile string
|
|
Root string
|
|
AutoRestart bool
|
|
Dns []string
|
|
DnsSearch []string
|
|
EnableIptables bool
|
|
EnableIpForward bool
|
|
DefaultIp net.IP
|
|
BridgeIface string
|
|
BridgeIP string
|
|
InterContainerCommunication bool
|
|
GraphDriver string
|
|
ExecDriver string
|
|
Mtu int
|
|
DisableNetwork bool
|
|
EnableSelinuxSupport bool
|
|
Context map[string][]string
|
|
}
|
|
|
|
// ConfigFromJob creates and returns a new DaemonConfig object
|
|
// by parsing the contents of a job's environment.
|
|
func ConfigFromJob(job *engine.Job) *Config {
|
|
config := &Config{
|
|
Pidfile: job.Getenv("Pidfile"),
|
|
Root: job.Getenv("Root"),
|
|
AutoRestart: job.GetenvBool("AutoRestart"),
|
|
EnableIptables: job.GetenvBool("EnableIptables"),
|
|
EnableIpForward: job.GetenvBool("EnableIpForward"),
|
|
BridgeIP: job.Getenv("BridgeIP"),
|
|
BridgeIface: job.Getenv("BridgeIface"),
|
|
DefaultIp: net.ParseIP(job.Getenv("DefaultIp")),
|
|
InterContainerCommunication: job.GetenvBool("InterContainerCommunication"),
|
|
GraphDriver: job.Getenv("GraphDriver"),
|
|
ExecDriver: job.Getenv("ExecDriver"),
|
|
EnableSelinuxSupport: job.GetenvBool("EnableSelinuxSupport"),
|
|
}
|
|
if dns := job.GetenvList("Dns"); dns != nil {
|
|
config.Dns = dns
|
|
}
|
|
if dnsSearch := job.GetenvList("DnsSearch"); dnsSearch != nil {
|
|
config.DnsSearch = dnsSearch
|
|
}
|
|
if mtu := job.GetenvInt("Mtu"); mtu != 0 {
|
|
config.Mtu = mtu
|
|
} else {
|
|
config.Mtu = GetDefaultNetworkMtu()
|
|
}
|
|
config.DisableNetwork = config.BridgeIface == DisableNetworkBridge
|
|
|
|
return config
|
|
}
|
|
|
|
func GetDefaultNetworkMtu() int {
|
|
if iface, err := networkdriver.GetDefaultRouteIface(); err == nil {
|
|
return iface.MTU
|
|
}
|
|
return defaultNetworkMtu
|
|
}
|