mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
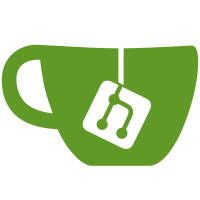
This makes separating middlewares from the core api easier. As an example, the authorization middleware is moved to it's own package. Initialize all static middlewares when the server is created, reducing allocations every time a route is wrapper with the middlewares. Signed-off-by: David Calavera <david.calavera@gmail.com>
37 lines
1.4 KiB
Go
37 lines
1.4 KiB
Go
package middleware
|
|
|
|
import (
|
|
"net/http"
|
|
|
|
"github.com/Sirupsen/logrus"
|
|
"golang.org/x/net/context"
|
|
)
|
|
|
|
// CORSMiddleware injects CORS headers to each request
|
|
// when it's configured.
|
|
type CORSMiddleware struct {
|
|
defaultHeaders string
|
|
}
|
|
|
|
// NewCORSMiddleware creates a new CORSMiddleware with default headers.
|
|
func NewCORSMiddleware(d string) CORSMiddleware {
|
|
return CORSMiddleware{defaultHeaders: d}
|
|
}
|
|
|
|
// WrapHandler returns a new handler function wrapping the previous one in the request chain.
|
|
func (c CORSMiddleware) WrapHandler(handler func(ctx context.Context, w http.ResponseWriter, r *http.Request, vars map[string]string) error) func(ctx context.Context, w http.ResponseWriter, r *http.Request, vars map[string]string) error {
|
|
return func(ctx context.Context, w http.ResponseWriter, r *http.Request, vars map[string]string) error {
|
|
// If "api-cors-header" is not given, but "api-enable-cors" is true, we set cors to "*"
|
|
// otherwise, all head values will be passed to HTTP handler
|
|
corsHeaders := c.defaultHeaders
|
|
if corsHeaders == "" {
|
|
corsHeaders = "*"
|
|
}
|
|
|
|
logrus.Debugf("CORS header is enabled and set to: %s", corsHeaders)
|
|
w.Header().Add("Access-Control-Allow-Origin", corsHeaders)
|
|
w.Header().Add("Access-Control-Allow-Headers", "Origin, X-Requested-With, Content-Type, Accept, X-Registry-Auth")
|
|
w.Header().Add("Access-Control-Allow-Methods", "HEAD, GET, POST, DELETE, PUT, OPTIONS")
|
|
return handler(ctx, w, r, vars)
|
|
}
|
|
}
|