mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
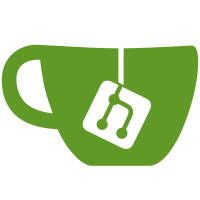
The libnetwork test does not need to run inside a namespace when inside a container. This results in unpredictable behavior when the sandbox code unlocks the go routine from the OS thread while the test code still wants it locked in the OS thread. This will result in unreachable interfaces when the go routine migrates to a different OS thread. Fixed by passing a special test flag which is only set to true when the test is run inside a container. Signed-off-by: Jana Radhakrishnan <mrjana@docker.com>
41 lines
942 B
Go
41 lines
942 B
Go
package netutils
|
|
|
|
import (
|
|
"flag"
|
|
"runtime"
|
|
"syscall"
|
|
"testing"
|
|
)
|
|
|
|
var runningInContainer = flag.Bool("incontainer", false, "Indicates if the test is running in a container")
|
|
|
|
// IsRunningInContainer returns whether the test is running inside a container.
|
|
func IsRunningInContainer() bool {
|
|
return (*runningInContainer)
|
|
}
|
|
|
|
// SetupTestNetNS joins a new network namespace, and returns its associated
|
|
// teardown function.
|
|
//
|
|
// Example usage:
|
|
//
|
|
// defer SetupTestNetNS(t)()
|
|
//
|
|
func SetupTestNetNS(t *testing.T) func() {
|
|
runtime.LockOSThread()
|
|
if err := syscall.Unshare(syscall.CLONE_NEWNET); err != nil {
|
|
t.Fatalf("Failed to enter netns: %v", err)
|
|
}
|
|
|
|
fd, err := syscall.Open("/proc/self/ns/net", syscall.O_RDONLY, 0)
|
|
if err != nil {
|
|
t.Fatal("Failed to open netns file")
|
|
}
|
|
|
|
return func() {
|
|
if err := syscall.Close(fd); err != nil {
|
|
t.Logf("Warning: netns closing failed (%v)", err)
|
|
}
|
|
runtime.UnlockOSThread()
|
|
}
|
|
}
|