mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
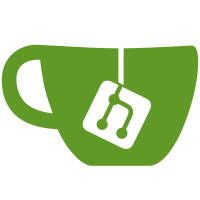
This fix is a follow up for comment: https://github.com/docker/docker/pull/28896#issuecomment-265392703 Currently secret name or ID prefix resolving is done at the client side, which means different behavior of API and CMD. This fix moves the resolving from client to daemon, with exactly the same rule: - Full ID - Full Name - Partial ID (prefix) All existing tests should pass. This fix is related to #288896, #28884 and may be related to #29125. Signed-off-by: Yong Tang <yong.tang.github@outlook.com>
41 lines
1.1 KiB
Go
41 lines
1.1 KiB
Go
package secret
|
|
|
|
import (
|
|
"github.com/docker/docker/cli"
|
|
"github.com/docker/docker/cli/command"
|
|
"github.com/docker/docker/cli/command/inspect"
|
|
"github.com/spf13/cobra"
|
|
"golang.org/x/net/context"
|
|
)
|
|
|
|
type inspectOptions struct {
|
|
names []string
|
|
format string
|
|
}
|
|
|
|
func newSecretInspectCommand(dockerCli *command.DockerCli) *cobra.Command {
|
|
opts := inspectOptions{}
|
|
cmd := &cobra.Command{
|
|
Use: "inspect [OPTIONS] SECRET [SECRET...]",
|
|
Short: "Display detailed information on one or more secrets",
|
|
Args: cli.RequiresMinArgs(1),
|
|
RunE: func(cmd *cobra.Command, args []string) error {
|
|
opts.names = args
|
|
return runSecretInspect(dockerCli, opts)
|
|
},
|
|
}
|
|
|
|
cmd.Flags().StringVarP(&opts.format, "format", "f", "", "Format the output using the given Go template")
|
|
return cmd
|
|
}
|
|
|
|
func runSecretInspect(dockerCli *command.DockerCli, opts inspectOptions) error {
|
|
client := dockerCli.Client()
|
|
ctx := context.Background()
|
|
|
|
getRef := func(id string) (interface{}, []byte, error) {
|
|
return client.SecretInspectWithRaw(ctx, id)
|
|
}
|
|
|
|
return inspect.Inspect(dockerCli.Out(), opts.names, opts.format, getRef)
|
|
}
|