mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
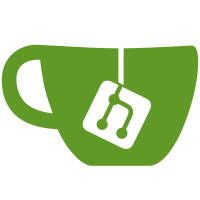
Use strongly typed errors to set HTTP status codes. Error interfaces are defined in the api/errors package and errors returned from controllers are checked against these interfaces. Errors can be wraeped in a pkg/errors.Causer, as long as somewhere in the line of causes one of the interfaces is implemented. The special error interfaces take precedence over Causer, meaning if both Causer and one of the new error interfaces are implemented, the Causer is not traversed. Signed-off-by: Brian Goff <cpuguy83@gmail.com>
86 lines
2 KiB
Go
86 lines
2 KiB
Go
package errdefs
|
|
|
|
type causer interface {
|
|
Cause() error
|
|
}
|
|
|
|
func getImplementer(err error) error {
|
|
switch e := err.(type) {
|
|
case
|
|
ErrNotFound,
|
|
ErrInvalidParameter,
|
|
ErrConflict,
|
|
ErrUnauthorized,
|
|
ErrUnavailable,
|
|
ErrForbidden,
|
|
ErrSystem,
|
|
ErrNotModified,
|
|
ErrNotImplemented,
|
|
ErrUnknown:
|
|
return e
|
|
case causer:
|
|
return getImplementer(e.Cause())
|
|
default:
|
|
return err
|
|
}
|
|
}
|
|
|
|
// IsNotFound returns if the passed in error is a ErrNotFound
|
|
func IsNotFound(err error) bool {
|
|
_, ok := getImplementer(err).(ErrNotFound)
|
|
return ok
|
|
}
|
|
|
|
// IsInvalidParameter returns if the passed in error is an ErrInvalidParameter
|
|
func IsInvalidParameter(err error) bool {
|
|
_, ok := getImplementer(err).(ErrInvalidParameter)
|
|
return ok
|
|
}
|
|
|
|
// IsConflict returns if the passed in error is a ErrConflict
|
|
func IsConflict(err error) bool {
|
|
_, ok := getImplementer(err).(ErrConflict)
|
|
return ok
|
|
}
|
|
|
|
// IsUnauthorized returns if the the passed in error is an ErrUnauthorized
|
|
func IsUnauthorized(err error) bool {
|
|
_, ok := getImplementer(err).(ErrUnauthorized)
|
|
return ok
|
|
}
|
|
|
|
// IsUnavailable returns if the passed in error is an ErrUnavailable
|
|
func IsUnavailable(err error) bool {
|
|
_, ok := getImplementer(err).(ErrUnavailable)
|
|
return ok
|
|
}
|
|
|
|
// IsForbidden returns if the passed in error is a ErrForbidden
|
|
func IsForbidden(err error) bool {
|
|
_, ok := getImplementer(err).(ErrForbidden)
|
|
return ok
|
|
}
|
|
|
|
// IsSystem returns if the passed in error is a ErrSystem
|
|
func IsSystem(err error) bool {
|
|
_, ok := getImplementer(err).(ErrSystem)
|
|
return ok
|
|
}
|
|
|
|
// IsNotModified returns if the passed in error is a NotModified error
|
|
func IsNotModified(err error) bool {
|
|
_, ok := getImplementer(err).(ErrNotModified)
|
|
return ok
|
|
}
|
|
|
|
// IsNotImplemented returns if the passed in error is a ErrNotImplemented
|
|
func IsNotImplemented(err error) bool {
|
|
_, ok := getImplementer(err).(ErrNotImplemented)
|
|
return ok
|
|
}
|
|
|
|
// IsUnknown returns if the passed in error is an ErrUnknown
|
|
func IsUnknown(err error) bool {
|
|
_, ok := getImplementer(err).(ErrUnknown)
|
|
return ok
|
|
}
|