mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
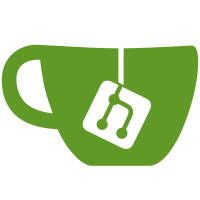
When plugins have a positive refcount, they were not allowed to be removed. However, plugins could still be disabled when volumes referenced it and containers using them were running. This change fixes that by enforcing plugin refcount during disable. A "force" disable option is also added to ignore reference refcounting. Signed-off-by: Anusha Ragunathan <anusha@docker.com>
69 lines
2.3 KiB
Go
69 lines
2.3 KiB
Go
// +build !linux
|
|
|
|
package plugin
|
|
|
|
import (
|
|
"errors"
|
|
"fmt"
|
|
"io"
|
|
"net/http"
|
|
|
|
"github.com/docker/docker/api/types"
|
|
"golang.org/x/net/context"
|
|
)
|
|
|
|
var errNotSupported = errors.New("plugins are not supported on this platform")
|
|
|
|
// Disable deactivates a plugin, which implies that they cannot be used by containers.
|
|
func (pm *Manager) Disable(name string, config *types.PluginDisableConfig) error {
|
|
return errNotSupported
|
|
}
|
|
|
|
// Enable activates a plugin, which implies that they are ready to be used by containers.
|
|
func (pm *Manager) Enable(name string, config *types.PluginEnableConfig) error {
|
|
return errNotSupported
|
|
}
|
|
|
|
// Inspect examines a plugin config
|
|
func (pm *Manager) Inspect(refOrID string) (tp types.Plugin, err error) {
|
|
// Even though plugin is not supported, we still want to return `not found`
|
|
// error so that `docker inspect` (without `--type` specified) returns correct
|
|
// `not found` message
|
|
return tp, fmt.Errorf("no such plugin name or ID associated with %q", refOrID)
|
|
}
|
|
|
|
// Privileges pulls a plugin config and computes the privileges required to install it.
|
|
func (pm *Manager) Privileges(name string, metaHeaders http.Header, authConfig *types.AuthConfig) (types.PluginPrivileges, error) {
|
|
return nil, errNotSupported
|
|
}
|
|
|
|
// Pull pulls a plugin, check if the correct privileges are provided and install the plugin.
|
|
func (pm *Manager) Pull(name string, metaHeader http.Header, authConfig *types.AuthConfig, privileges types.PluginPrivileges) error {
|
|
return errNotSupported
|
|
}
|
|
|
|
// List displays the list of plugins and associated metadata.
|
|
func (pm *Manager) List() ([]types.Plugin, error) {
|
|
return nil, errNotSupported
|
|
}
|
|
|
|
// Push pushes a plugin to the store.
|
|
func (pm *Manager) Push(name string, metaHeader http.Header, authConfig *types.AuthConfig) error {
|
|
return errNotSupported
|
|
}
|
|
|
|
// Remove deletes plugin's root directory.
|
|
func (pm *Manager) Remove(name string, config *types.PluginRmConfig) error {
|
|
return errNotSupported
|
|
}
|
|
|
|
// Set sets plugin args
|
|
func (pm *Manager) Set(name string, args []string) error {
|
|
return errNotSupported
|
|
}
|
|
|
|
// CreateFromContext creates a plugin from the given pluginDir which contains
|
|
// both the rootfs and the config.json and a repoName with optional tag.
|
|
func (pm *Manager) CreateFromContext(ctx context.Context, tarCtx io.Reader, options *types.PluginCreateOptions) error {
|
|
return errNotSupported
|
|
}
|