mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
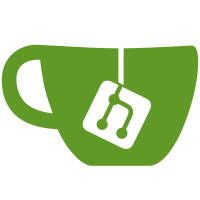
Save was failing file integrity checksums due to bugs in both Windows and Docker. This commit includes fixes to file time handling in tarexport and system.chtimes that are necessary along with the Windows platform fixes to correctly support save. With this change, sysfile_backups for windowsfilter driver are no longer needed, so that code is removed. Signed-off-by: Stefan J. Wernli <swernli@microsoft.com>
52 lines
1.1 KiB
Go
52 lines
1.1 KiB
Go
package system
|
|
|
|
import (
|
|
"os"
|
|
"syscall"
|
|
"time"
|
|
"unsafe"
|
|
)
|
|
|
|
var (
|
|
maxTime time.Time
|
|
)
|
|
|
|
func init() {
|
|
if unsafe.Sizeof(syscall.Timespec{}.Nsec) == 8 {
|
|
// This is a 64 bit timespec
|
|
// os.Chtimes limits time to the following
|
|
maxTime = time.Unix(0, 1<<63-1)
|
|
} else {
|
|
// This is a 32 bit timespec
|
|
maxTime = time.Unix(1<<31-1, 0)
|
|
}
|
|
}
|
|
|
|
// Chtimes changes the access time and modified time of a file at the given path
|
|
func Chtimes(name string, atime time.Time, mtime time.Time) error {
|
|
unixMinTime := time.Unix(0, 0)
|
|
unixMaxTime := maxTime
|
|
|
|
// If the modified time is prior to the Unix Epoch, or after the
|
|
// end of Unix Time, os.Chtimes has undefined behavior
|
|
// default to Unix Epoch in this case, just in case
|
|
|
|
if atime.Before(unixMinTime) || atime.After(unixMaxTime) {
|
|
atime = unixMinTime
|
|
}
|
|
|
|
if mtime.Before(unixMinTime) || mtime.After(unixMaxTime) {
|
|
mtime = unixMinTime
|
|
}
|
|
|
|
if err := os.Chtimes(name, atime, mtime); err != nil {
|
|
return err
|
|
}
|
|
|
|
// Take platform specific action for setting create time.
|
|
if err := setCTime(name, mtime); err != nil {
|
|
return err
|
|
}
|
|
|
|
return nil
|
|
}
|