mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
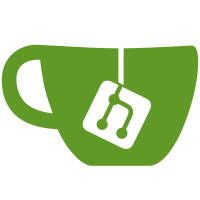
- some method names were changed to have a 'Locking' suffix, as the downcased versions already existed, and the existing functions simply had locks around the already downcased version. - deleting unused functions - package comment - magic numbers replaced by golang constants - comments all over Signed-off-by: Morgan Bauer <mbauer@us.ibm.com>
32 lines
671 B
Go
32 lines
671 B
Go
package daemon
|
|
|
|
import (
|
|
"sort"
|
|
)
|
|
|
|
// History is a convenience type for storing a list of containers,
|
|
// ordered by creation date.
|
|
type History []*Container
|
|
|
|
func (history *History) Len() int {
|
|
return len(*history)
|
|
}
|
|
|
|
func (history *History) Less(i, j int) bool {
|
|
containers := *history
|
|
return containers[j].Created.Before(containers[i].Created)
|
|
}
|
|
|
|
func (history *History) Swap(i, j int) {
|
|
containers := *history
|
|
containers[i], containers[j] = containers[j], containers[i]
|
|
}
|
|
|
|
// Add the given container to history.
|
|
func (history *History) Add(container *Container) {
|
|
*history = append(*history, container)
|
|
}
|
|
|
|
func (history *History) sort() {
|
|
sort.Sort(history)
|
|
}
|