mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
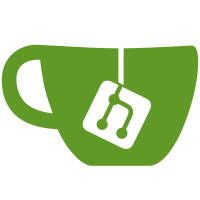
Fix for #13175. This change allows user-input timestamps (e.g. to `docker events --since/--until` or `docker logs --since` to be parsed using standard RFC3339Nano layout in Go instead of the layout that parses all timestamps into fixed-length strings (currently buggy). User inputs need not to be complying to the internal format (`RFC3339NanoFixed`) anyway. Added test case for `events --since/--until` with all possible timestamp input formats. Signed-off-by: Ahmet Alp Balkan <ahmetalpbalkan@gmail.com>
29 lines
646 B
Go
29 lines
646 B
Go
package timeutils
|
|
|
|
import (
|
|
"strconv"
|
|
"strings"
|
|
"time"
|
|
)
|
|
|
|
// GetTimestamp tries to parse given string as RFC3339 time
|
|
// or Unix timestamp (with seconds precision), if successful
|
|
//returns a Unix timestamp as string otherwise returns value back.
|
|
func GetTimestamp(value string) string {
|
|
var format string
|
|
if strings.Contains(value, ".") {
|
|
format = time.RFC3339Nano
|
|
} else {
|
|
format = time.RFC3339
|
|
}
|
|
|
|
loc := time.FixedZone(time.Now().Zone())
|
|
if len(value) < len(format) {
|
|
format = format[:len(value)]
|
|
}
|
|
t, err := time.ParseInLocation(format, value, loc)
|
|
if err != nil {
|
|
return value
|
|
}
|
|
return strconv.FormatInt(t.Unix(), 10)
|
|
}
|