mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
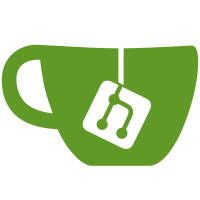
Layer store manages read-only and read-write layers on a union file system. Read only layers are always referenced by content addresses. Read-write layer identifiers are handled by the caller but upon registering its difference, the committed read-only layer will be referenced by content hash. Signed-off-by: Derek McGowan <derek@mcgstyle.net> (github: dmcgowan) Signed-off-by: Tonis Tiigi <tonistiigi@gmail.com>
64 lines
1.2 KiB
Go
64 lines
1.2 KiB
Go
package layer
|
|
|
|
import "io"
|
|
|
|
type mountedLayer struct {
|
|
name string
|
|
mountID string
|
|
initID string
|
|
parent *roLayer
|
|
path string
|
|
layerStore *layerStore
|
|
activityCount int
|
|
}
|
|
|
|
func (ml *mountedLayer) cacheParent() string {
|
|
if ml.initID != "" {
|
|
return ml.initID
|
|
}
|
|
if ml.parent != nil {
|
|
return ml.parent.cacheID
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (ml *mountedLayer) TarStream() (io.Reader, error) {
|
|
archiver, err := ml.layerStore.driver.Diff(ml.mountID, ml.cacheParent())
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return autoClosingReader{archiver}, nil
|
|
}
|
|
|
|
func (ml *mountedLayer) Path() (string, error) {
|
|
if ml.path == "" {
|
|
return "", ErrNotMounted
|
|
}
|
|
return ml.path, nil
|
|
}
|
|
|
|
func (ml *mountedLayer) Parent() Layer {
|
|
if ml.parent != nil {
|
|
return ml.parent
|
|
}
|
|
|
|
// Return a nil interface instead of an interface wrapping a nil
|
|
// pointer.
|
|
return nil
|
|
}
|
|
|
|
func (ml *mountedLayer) Size() (int64, error) {
|
|
return ml.layerStore.driver.DiffSize(ml.mountID, ml.cacheParent())
|
|
}
|
|
|
|
type autoClosingReader struct {
|
|
source io.ReadCloser
|
|
}
|
|
|
|
func (r autoClosingReader) Read(p []byte) (n int, err error) {
|
|
n, err = r.source.Read(p)
|
|
if err != nil {
|
|
r.source.Close()
|
|
}
|
|
return
|
|
}
|